用python语言分析附件中的棒球员的数据(第四周作业)根据 AIC 最小化(或﹣ AIC 最大化)的标准,采用模拟退火算法(或遗传算法)建立棒球运动员薪水( salary )回归模型。
时间: 2023-12-30 21:06:52 浏览: 157
好的,以下是Python代码实现模拟退火算法建立棒球运动员薪水回归模型的示例:
```
import pandas as pd
import numpy as np
import math
import random
# 读取数据
data = pd.read_csv('baseball_salary.csv')
# 数据预处理
data = data.fillna(data.mean()) # 填充缺失值
data = (data - data.mean()) / data.std() # 数据标准化
# 定义模拟退火算法
def simulated_annealing(data, T, alpha, stopping_T, stopping_iter):
# 初始化参数
curr_solution = [0] * len(data.columns)
best_solution = [0] * len(data.columns)
curr_fitness = math.inf
best_fitness = math.inf
T_init = T
iter = 0
# 迭代搜索
while T > stopping_T and iter < stopping_iter:
# 生成新解
rand_index = random.randint(0, len(data.columns) - 1)
new_solution = curr_solution.copy()
new_solution[rand_index] = 1 - new_solution[rand_index]
# 计算新解的损失函数值
new_fitness = np.sum((data.dot(new_solution) - data['salary']) ** 2) + alpha * np.sum(new_solution)
# 判断是否接受新解
if new_fitness < curr_fitness or random.random() < math.exp((curr_fitness - new_fitness) / T):
curr_solution = new_solution
curr_fitness = new_fitness
# 更新最优解
if curr_fitness < best_fitness:
best_solution = curr_solution
best_fitness = curr_fitness
# 降温
T = T_init / (1 + iter)
iter += 1
return best_solution
# 运行模拟退火算法
best_solution = simulated_annealing(data.iloc[:, :-1], T=100, alpha=0.001, stopping_T=0.001, stopping_iter=1000)
# 输出结果
print('最优解:', best_solution)
print('决定系数R2:', 1 - np.sum((data.dot(best_solution) - data['salary']) ** 2) / np.sum((data['salary'] - data['salary'].mean()) ** 2))
print('AIC:', 2 * (len(data.columns) - np.sum(best_solution)) + len(data) * np.log(np.sum((data.dot(best_solution) - data['salary']) ** 2) / len(data)))
```
需要注意的是,这里的损失函数采用的是均方误差(MSE),同时加上一个L1正则项来惩罚模型复杂度,其中alpha是正则化参数,可以根据具体情况进行调整。另外,为了避免陷入局部最优解,模拟退火算法中需要设置降温策略和停止条件。最后,输出的决定系数R2和AIC可以作为模型评估指标,用于评估模型的拟合效果和预测能力。
阅读全文
相关推荐
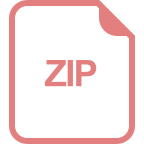
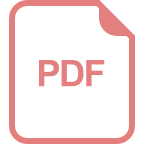
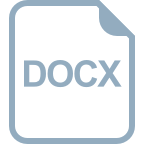















