用Java设计一个名为MyCircle的类。要求定义MyCircle类,根据题目要求定义属性和方法,检验程序是否正确
时间: 2024-09-18 19:15:55 浏览: 25
在Java中,我们可以设计一个名为`MyCircle`的类来表示一个圆,它通常需要定义属性如半径`radius`,以及一些常用的方法,例如计算圆的周长、面积、以及判断一个给定点是否在圆内等。这里是一个基本的设计:
```java
public class MyCircle {
// 圆的半径
private double radius;
// 构造函数接受半径作为参数
public MyCircle(double radius) {
if (radius <= 0) {
throw new IllegalArgumentException("Radius must be positive");
}
this.radius = radius;
}
// Getter and Setter for radius
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
// 计算圆的周长
public double circumference() {
return Math.PI * 2 * radius;
}
// 计算圆的面积
public double area() {
return Math.PI * radius * radius;
}
// 判断一个点(坐标x, y)是否在圆内
public boolean isPointInside(int x, int y) {
int distance = (int) Math.pow(x - getCenterX(), 2) + (int) Math.pow(y - getCenterY(), 2);
return distance < (int) Math.pow(radius, 2); // 这里简化处理,实际应用中应使用Math.sqrt
}
// 获取圆心的x坐标,因为我们默认圆心在原点,所以返回0
private double getCenterX() {
return 0;
}
// 获取圆心的y坐标,同理
private double getCenterY() {
return 0;
}
// toString方法便于打印圆的相关信息
@Override
public String toString() {
return "MyCircle[radius=" + radius + "]";
}
}
```
这个`MyCircle`类满足了题目的要求,但是要注意在实际使用中,`isPointInside`方法的判断可能会更复杂,因为需要考虑浮点数精度问题。此外,如果你希望圆心不是固定在原点,你需要额外定义`centerX`和`centerY`属性。
相关推荐
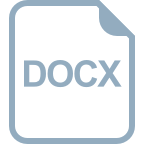
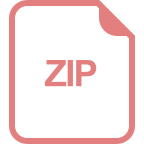














