写一个yolo标签旋转转换的python代码
时间: 2024-01-13 11:05:53 浏览: 26
以下是一个基于OpenCV的Python代码实现YOLO标签旋转转换:
```python
import cv2
import numpy as np
def rotate_yolo_label(label_path, image_path, angle):
# 读取图像和标签文件
img = cv2.imread(image_path)
label_file = open(label_path, 'r')
label_lines = label_file.readlines()
label_file.close()
# 获取图像宽度和高度
img_height, img_width, _ = img.shape
# 逐行读取标签文件并旋转坐标
result = []
for line in label_lines:
line = line.strip().split()
class_id = line[0]
x_center = float(line[1])
y_center = float(line[2])
width = float(line[3])
height = float(line[4])
# 将YOLO格式坐标转换为绝对坐标
x1 = int((x_center - width / 2) * img_width)
y1 = int((y_center - height / 2) * img_height)
x2 = int((x_center + width / 2) * img_width)
y2 = int((y_center + height / 2) * img_height)
# 构建旋转矩阵
rotate_matrix = cv2.getRotationMatrix2D((img_width/2, img_height/2), angle, 1)
# 旋转坐标点
rotated_points = np.dot(rotate_matrix, np.array([[x1, y1, 1], [x2, y2, 1]], dtype=np.float32).T)
rotated_points = rotated_points.T
# 将坐标点转换为YOLO格式
x_center = (rotated_points[0][0] + rotated_points[1][0]) / 2 / img_width
y_center = (rotated_points[0][1] + rotated_points[1][1]) / 2 / img_height
width = abs(rotated_points[0][0] - rotated_points[1][0]) / img_width
height = abs(rotated_points[0][1] - rotated_points[1][1]) / img_height
# 将旋转后的标签信息添加到结果列表中
result.append(f"{class_id} {x_center} {y_center} {width} {height}\n")
# 将旋转后的标签信息写入到文件中
with open(label_path, 'w') as f:
f.writelines(result)
# 旋转图像
rotated_img = cv2.warpAffine(img, rotate_matrix, (img_width, img_height))
# 返回旋转后的图像和标签
return rotated_img, label_path
```
该函数需要传入三个参数:标签文件路径、图像文件路径和旋转角度。函数会读取标签文件中的每一行信息,将YOLO格式坐标转换为绝对坐标,然后通过旋转矩阵将坐标点进行旋转,并将旋转后的坐标点转换为YOLO格式,最后将旋转后的标签信息写入到文件中。同时,函数也会通过OpenCV库将图像进行旋转,并返回旋转后的图像和标签文件路径。
相关推荐
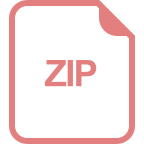










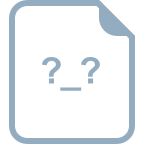
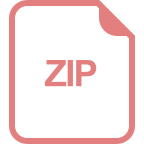
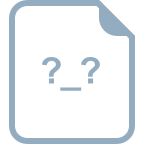
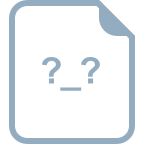
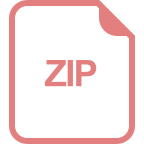