写一个随机生成旋转角度图片更改YOLO标签转换的程序
时间: 2023-12-10 16:02:35 浏览: 31
好的,以下是一个随机生成旋转角度图片更改YOLO标签转换的程序示例代码:
```python
import cv2
import numpy as np
import random
# 定义旋转函数
def rotate(image, angle):
h, w = image.shape[:2]
center = (w / 2, h / 2)
M = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated = cv2.warpAffine(image, M, (w, h))
return rotated, M
# 定义YOLO标签转换函数
def convert_yolo_label(label, angle, image_size):
# 解析标签
class_id, x, y, w, h = label.split()
# 计算旋转后的坐标
x, y = float(x), float(y)
w, h = float(w), float(h)
x1, y1 = x - w / 2, y - h / 2
x2, y2 = x + w / 2, y - h / 2
x3, y3 = x + w / 2, y + h / 2
x4, y4 = x - w / 2, y + h / 2
points = np.array([[x1, y1], [x2, y2], [x3, y3], [x4, y4]])
rot_mat = cv2.getRotationMatrix2D((x * image_size[0], y * image_size[1]), angle, 1)
points = np.dot(rot_mat, np.vstack((points.T, np.ones(4))))
x1, y1 = points[0][0] / image_size[0], points[1][0] / image_size[1]
x2, y2 = points[0][1] / image_size[0], points[1][1] / image_size[1]
x3, y3 = points[0][2] / image_size[0], points[1][2] / image_size[1]
x4, y4 = points[0][3] / image_size[0], points[1][3] / image_size[1]
x, y = (x1 + x3) / 2, (y1 + y3) / 2
w, h = np.abs(x1 - x3), np.abs(y1 - y3)
# 返回旋转后的标签
return f"{class_id} {x} {y} {w} {h}"
# 加载图像和标签
image = cv2.imread("image.jpg")
labels = ["0 0.5 0.5 0.3 0.4", "1 0.2 0.7 0.4 0.2"]
# 随机生成旋转角度
angle = random.randint(0, 360)
# 旋转图像和标签
rotated_image, M = rotate(image, angle)
rotated_labels = [convert_yolo_label(label, angle, image.shape[:2]) for label in labels]
# 显示旋转后的图像和标签
cv2.imshow("Rotated Image", rotated_image)
print("Rotated Labels:")
for label in rotated_labels:
print(label)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该程序与前一个示例程序非常相似,不同之处在于我们现在使用随机生成的旋转角度来旋转图像和标签,而不是在代码中硬编码旋转角度。
我们还将转换YOLO标签的函数修改为包括图像大小作为参数,并使用图像大小将标签坐标转换为比例。这样,我们就可以在任何大小的图像上使用该函数。
请注意,此代码仅供参考。在实际使用中,您可能需要根据您的数据集格式和需求进行修改。
相关推荐
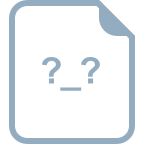
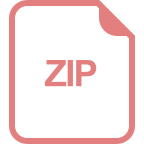














