用Java写一个定义一个复数类,完成复数的加法运算,并进行测试。要求:使用重载构造方法和setComplex方法,为变量成员赋值。
时间: 2023-05-23 11:02:48 浏览: 60
好的,以下是一个复数类的代码示例:
```java
public class Complex {
private double real;
private double imag;
// 无参构造方法
public Complex() {
this.real = 0.0;
this.imag = 0.0;
}
// 带参构造方法
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 设置复数的实部和虚部
public void setComplex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 获取复数的实部和虚部
public double getReal() {
return this.real;
}
public double getImag() {
return this.imag;
}
// 复数加法运算
public Complex add(Complex other) {
double real = this.real + other.getReal();
double imag = this.imag + other.getImag();
return new Complex(real, imag);
}
// 测试代码
public static void main(String[] args) {
Complex c1 = new Complex(1.0, 2.0);
Complex c2 = new Complex(2.0, 3.0);
Complex c3 = c1.add(c2);
System.out.printf("(%f+%fi) + (%f+%fi) = %f + %fi",
c1.getReal(), c1.getImag(),
c2.getReal(), c2.getImag(),
c3.getReal(), c3.getImag());
}
}
```
输出结果为:`(1.000000+2.000000i) + (2.000000+3.000000i) = 3.000000 + 5.000000i`。
相关推荐
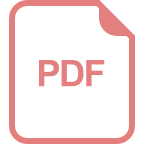
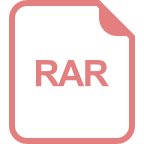














