int CountLeaf(BiTree T, int& count) { if (T == NULL) { return 0; } if (T->left == NULL && T->right == NULL) { count++; } CountLeaf(T->left, count); CountLeaf(T->right, count); return count; } void main() { BiTree(T); int i=1,j=1,choice; while(j) { printf("\n"); printf("\n\t\t"); printf("\n\t\t***************************************"); printf("\n\t\t* 1-------初始化 *"); printf("\n\t\t* 2-------输出中序遍历结果 *"); printf("\n\t\t* 3-------输出前序 *"); printf("\n\t\t* 4-------输出后序 *"); printf("\n\t\t* 5-------计算二叉树的深度 *"); printf("\n\t\t* 6-------统计二叉数的结点个数 *"); printf("\n\t\t* 7-------统计二叉树的叶结点个数 *"); printf("\n\t\t* 0-------返回 *"); printf("\n\t\t***************************************"); printf("\n请选择菜单号(0---7):"); scanf("%d",&choice); switch(choice) { case 1:InOrderTraverse(T); break; case 2:Preorder_Traversal(T); break; case 3:Postorder_Traversal(T); break; case 4:Depth(T); break; case 5:NodeCount(T); break; case 6:CountLeaf(T); break; case 7: j=0; break; } } }
时间: 2023-07-01 19:16:26 浏览: 67
这段代码是一个二叉树的程序,主要实现了以下功能:
1. 初始化二叉树(不在代码中显示);
2. 输出中序遍历结果;
3. 输出前序遍历结果;
4. 输出后序遍历结果;
5. 计算二叉树的深度;
6. 统计二叉树的结点个数;
7. 统计二叉树的叶结点个数。
其中,函数 CountLeaf 实现了统计二叉树的叶结点个数的功能。该函数采用了递归的方式,先判断当前节点是否为叶子结点,如果是,则计数器 count 加一;然后递归地处理左子树和右子树。最后返回叶子结点的个数。值得注意的是,函数 CountLeaf 的第二个参数 count 是一个引用类型,因为函数内部需要更新 count 的值。
函数 main 实现了一个简单的交互式菜单,可以让用户选择需要执行的功能。用户可以通过输入菜单号来选择相应的功能。
相关问题
#include<stdio.h> #include<iostream.h> #include<stdlib.h> typedef char ElementType; typedef struct TNode { ElementType Data; struct TNode *left, *right; }TNode, *BiTree; void InOrderTraverse(BiTree T) { if(T) { InOrderTraverse(T->left); cout<<T->Data; InOrderTraverse(T->right); } } void Preorder_Traversal(BiTree T) { if(!T)return; cout<<T->Data; Preorder_Traversal(T->left); Preorder_Traversal(T->right); } void Postorder_Traversal(BiTree T) { if(!T)return; Postorder_Traversal(T->left); Postorder_Traversal(T->right); cout<<T->Data; } int Depth(BiTree T) { int m,n; if(T==NULL) return 0; else { m=Depth(T->left); n=Depth(T->right); if(m>n) return(m+1); else return(n+1); } } int NodeCount(BiTree T) { if(T==NULL) return 0; else return NodeCount(T->left)+NodeCount(T->right)+1; } int CountLeaf(BiTree* T, int* count) { if (T!=NULL&&(T->left)== NULL&&(T->right)==NULL) { (*count)++; } if (T!= NULL) { CountLeaf(T->left, count); CountLeaf(T->reght, count); } return *count; }
这段代码有很多问题,我来一一指出:
1. `#include<iostream.h>` 应该改为 `#include<iostream>`,因为 `<iostream.h>` 是旧版 C++ 标准库,现在已经被弃用。
2. 在 `CountLeaf` 函数中,`T` 是一个指向指针的指针,应该改为 `BiTree T`。
3. 在 `CountLeaf` 函数中,`T->reght` 应该改为 `T->right`。
4. 在 `CountLeaf` 函数中,`count` 是一个指向 `int` 类型的指针,应该改为 `int& count`,这样才能在递归中正确累加叶子结点的数量。
5. 在 `CountLeaf` 函数中,应该在最开始将 `*count` 初始化为 0,否则会返回一个随机值。
6. 在 `CountLeaf` 函数中,应该在递归结束后返回累加后的叶子结点数量,否则会返回一个随机值。
下面是修改后的代码:
```c++
#include <stdio.h>
#include <iostream>
#include <stdlib.h>
using namespace std;
typedef char ElementType;
typedef struct TNode
{
ElementType Data;
struct TNode *left, *right;
}TNode, *BiTree;
void InOrderTraverse(BiTree T)
{
if(T)
{
InOrderTraverse(T->left);
cout<<T->Data;
InOrderTraverse(T->right);
}
}
void Preorder_Traversal(BiTree T)
{
if(!T)return;
cout<<T->Data;
Preorder_Traversal(T->left);
Preorder_Traversal(T->right);
}
void Postorder_Traversal(BiTree T)
{
if(!T)return;
Postorder_Traversal(T->left);
Postorder_Traversal(T->right);
cout<<T->Data;
}
int Depth(BiTree T)
{
int m,n;
if(T==NULL) return 0;
else
{
m=Depth(T->left);
n=Depth(T->right);
if(m>n)
return(m+1);
else
return(n+1);
}
}
int NodeCount(BiTree T)
{
if(T==NULL)
return 0;
else
return NodeCount(T->left)+NodeCount(T->right)+1;
}
int CountLeaf(BiTree T, int& count)
{
if (T == NULL) {
return 0;
}
if (T->left == NULL && T->right == NULL) {
count++;
}
CountLeaf(T->left, count);
CountLeaf(T->right, count);
return count;
}
int main()
{
BiTree T = new TNode;
T->Data = 'A';
T->left = new TNode;
T->left->Data = 'B';
T->left->left = NULL;
T->left->right = NULL;
T->right = new TNode;
T->right->Data = 'C';
T->right->left = new TNode;
T->right->left->Data = 'D';
T->right->left->left = NULL;
T->right->left->right = NULL;
T->right->right = new TNode;
T->right->right->Data = 'E';
T->right->right->left = NULL;
T->right->right->right = NULL;
cout << "Inorder traversal: ";
InOrderTraverse(T);
cout << endl;
cout << "Preorder traversal: ";
Preorder_Traversal(T);
cout << endl;
cout << "Postorder traversal: ";
Postorder_Traversal(T);
cout << endl;
cout << "Depth: " << Depth(T) << endl;
cout << "Node count: " << NodeCount(T) << endl;
int count = 0;
cout << "Leaf count: " << CountLeaf(T, count) << endl;
return 0;
}
```
#include <stdio.h> #include <stdlib.h> typedef struct TreeNode { char data; struct TreeNode *left; struct TreeNode *right; } TreeNode; TreeNode* createTree() { char ch; scanf("%c", &ch); if (ch == ' ') { return NULL; } TreeNode *node = (TreeNode*)malloc(sizeof(TreeNode)); node->data = ch; node->left = createTree(); node->right = createTree(); return node; } int countLeaf(TreeNode *root) { if (root == NULL) { return 0; } if (root->left == NULL && root->right == NULL) { return 1; } return countLeaf(root->left) + countLeaf(root->right); } int getHeight(TreeNode *root) { if (root == NULL) { return 0; } int leftHeight = getHeight(root->left); int rightHeight = getHeight(root->right); return (leftHeight > rightHeight ? leftHeight : rightHeight) + 1; } int main() { printf("请输入先序序列:\n"); TreeNode *root = createTree(); printf("叶结点个数:%d\n", countLeaf(root)); printf("树的高度:%d\n", getHeight(root)); return 0;标一下注释
以下是对代码的注释:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义树的结点结构体
typedef struct TreeNode {
char data; // 数据域
struct TreeNode *left; // 左子树指针
struct TreeNode *right; // 右子树指针
} TreeNode;
// 创建树的函数,利用先序遍历的方式输入树的结点
TreeNode* createTree() {
char ch;
scanf("%c", &ch); // 读入字符
if (ch == ' ') { // 如果当前字符为空格,则返回 NULL
return NULL;
}
TreeNode *node = (TreeNode*)malloc(sizeof(TreeNode)); // 创建树的结点
node->data = ch; // 将字符赋值给数据域
node->left = createTree(); // 递归创建左子树
node->right = createTree(); // 递归创建右子树
return node; // 返回树的结点指针
}
// 计算树的叶子结点个数的函数
int countLeaf(TreeNode *root) {
if (root == NULL) { // 如果当前结点为空,则返回 0
return 0;
}
if (root->left == NULL && root->right == NULL) { // 如果当前结点没有左右子树,则为叶子结点,返回 1
return 1;
}
return countLeaf(root->left) + countLeaf(root->right); // 递归计算左右子树的叶子结点个数并相加,返回结果
}
// 计算树的高度的函数
int getHeight(TreeNode *root) {
if (root == NULL) { // 如果当前结点为空,则返回 0
return 0;
}
int leftHeight = getHeight(root->left); // 计算左子树的高度
int rightHeight = getHeight(root->right); // 计算右子树的高度
return (leftHeight > rightHeight ? leftHeight : rightHeight) + 1; // 返回左右子树高度的较大值加1
}
int main() {
printf("请输入先序序列:\n");
TreeNode *root = createTree(); // 创建树
printf("叶结点个数:%d\n", countLeaf(root)); // 输出叶子结点个数
printf("树的高度:%d\n", getHeight(root)); // 输出树的高度
return 0;
}
```
相关推荐
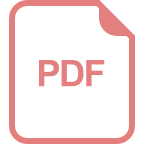













