写一段神经网络代码,注释写详细
时间: 2023-11-14 22:08:16 浏览: 39
以下是一个简单的神经网络代码,实现了一个两层的前向传播过程,其中包括输入层、隐藏层和输出层。注释包含了详细的说明,帮助理解代码实现的过程。
```python
import numpy as np
# 定义输入数据
X = np.array([[0, 0, 1],
[0, 1, 1],
[1, 0, 1],
[1, 1, 1]])
# 定义标签数据
y = np.array([[0],
[1],
[1],
[0]])
# 设置随机种子,确保每次运行结果一致
np.random.seed(1)
# 初始化权重参数,W1表示从输入层到隐藏层的权重,W2表示从隐藏层到输出层的权重
W1 = np.random.randn(3, 4)
W2 = np.random.randn(4, 1)
# 定义激活函数sigmoid
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义前向传播过程
def forward_propagation(X, W1, W2):
# 计算隐藏层的输入
z1 = np.dot(X, W1)
# 计算隐藏层的输出
a1 = sigmoid(z1)
# 计算输出层的输入
z2 = np.dot(a1, W2)
# 计算输出层的输出
a2 = sigmoid(z2)
return a1, a2
# 定义损失函数
def compute_cost(y, y_hat):
# 计算交叉熵损失
cost = -np.sum(y * np.log(y_hat) + (1 - y) * np.log(1 - y_hat))
return cost
# 定义反向传播过程
def backward_propagation(X, y, a1, a2, W1, W2):
# 计算输出层的误差
delta2 = (a2 - y) * a2 * (1 - a2)
# 计算隐藏层的误差
delta1 = np.dot(delta2, W2.T) * a1 * (1 - a1)
# 更新权重参数
W2 -= np.dot(a1.T, delta2)
W1 -= np.dot(X.T, delta1)
return W1, W2
# 训练模型
for i in range(10000):
# 前向传播
a1, a2 = forward_propagation(X, W1, W2)
# 计算损失
cost = compute_cost(y, a2)
# 反向传播
W1, W2 = backward_propagation(X, y, a1, a2, W1, W2)
# 每1000次迭代输出一次损失
if i % 1000 == 0:
print("Iteration: %d, Cost: %.4f" % (i, cost))
```
该代码实现了一个简单的两层神经网络,其中输入层有3个节点,输出层有1个节点,隐藏层有4个节点。使用sigmoid作为激活函数,并使用交叉熵作为损失函数。在训练过程中,使用反向传播算法更新权重参数,以最小化损失函数。
相关推荐
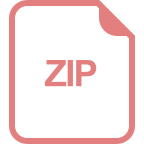
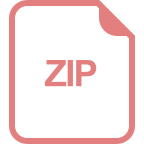
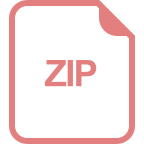














