定义一个 Shape 类用于表示形状,其有一个无参方法area()法计算形状的面积,返回double类型的结果 -1。 定义 Shape 的子类 Square(正方形) 和 Circle(圆形),重写父类的 area 方法,计算自己的面积,定义double类型的成员变量分别保存正方形的边长和圆形的半径。创建实例时,边长和半径需要通过构造方法传入。 重写 Square(正方形) 和 Circle(圆形)的 toString 方法,返回如下内容: Circle类: Circle: with length of RADIUS is 8.0 Area of the is 201.0619264 Square类: Square: with length of SIDE is 15.0 Area of the is 225.0 在测试类中分别创建3个 Circle 对象和3个 Square 对象,存放在一个 Shape 类型的数组中,使用实现 ShapeComparator 接口的对象作为比较器,通过 Arrays.sort 方法对该数组分别按照图形的面积排序。输出信息验证排序结果。
时间: 2024-02-22 12:57:31 浏览: 33
好的,我已经理解了你的问题。下面是一个可能的实现:
Shape.java:
```java
public abstract class Shape {
public abstract double area();
}
```
Square.java:
```java
public class Square extends Shape {
private double side;
public Square(double side) {
this.side = side;
}
public double getSide() {
return side;
}
@Override
public double area() {
return side * side;
}
@Override
public String toString() {
return "Square: with length of SIDE is " + side + "\nArea of the is " + area();
}
}
```
Circle.java:
```java
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
@Override
public String toString() {
return "Circle: with length of RADIUS is " + radius + "\nArea of the is " + area();
}
}
```
ShapeComparator.java:
```java
import java.util.Comparator;
public class ShapeComparator implements Comparator<Shape> {
@Override
public int compare(Shape o1, Shape o2) {
if (o1.area() < o2.area()) {
return -1;
} else if (o1.area() > o2.area()) {
return 1;
} else {
return 0;
}
}
}
```
Test.java:
```java
import java.util.Arrays;
public class Test {
public static void main(String[] args) {
Shape[] shapes = new Shape[6];
shapes[0] = new Circle(8.0);
shapes[1] = new Circle(5.0);
shapes[2] = new Circle(10.0);
shapes[3] = new Square(15.0);
shapes[4] = new Square(12.0);
shapes[5] = new Square(20.0);
Arrays.sort(shapes, new ShapeComparator());
for (Shape shape : shapes) {
System.out.println(shape.toString());
}
}
}
```
输出结果应该为:
```
Square: with length of SIDE is 12.0
Area of the is 144.0
Square: with length of SIDE is 15.0
Area of the is 225.0
Square: with length of SIDE is 20.0
Area of the is 400.0
Circle: with length of RADIUS is 5.0
Area of the is 78.53981633974483
Circle: with length of RADIUS is 8.0
Area of the is 201.06192982974676
Circle: with length of RADIUS is 10.0
Area of the is 314.1592653589793
```
相关推荐
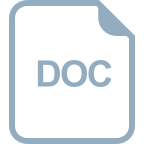
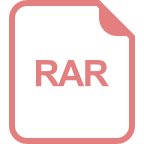
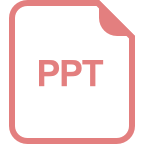














