写一个python扫雷游戏
时间: 2023-07-04 18:05:46 浏览: 37
好的,以下是一个简单的Python扫雷游戏:
```python
import random
class Minesweeper:
def __init__(self, width, height, mines):
self.width = width
self.height = height
self.mines = mines
self.game_over = False
self.board = [[0 for _ in range(width)] for _ in range(height)]
self.generate_board()
def generate_board(self):
# randomly place mines on board
placed_mines = 0
while placed_mines < self.mines:
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
if self.board[y][x] == 0:
self.board[y][x] = "*"
placed_mines += 1
# fill in numbers for non-mine cells
for y in range(self.height):
for x in range(self.width):
if self.board[y][x] == "*":
continue
neighbors = self.get_neighbors(x, y)
num_mines = sum([1 for nx, ny in neighbors if self.board[ny][nx] == "*"])
self.board[y][x] = num_mines
def get_neighbors(self, x, y):
neighbors = []
for dx in [-1, 0, 1]:
for dy in [-1, 0, 1]:
if dx == 0 and dy == 0:
continue
nx = x + dx
ny = y + dy
if nx < 0 or nx >= self.width or ny < 0 or ny >= self.height:
continue
neighbors.append((nx, ny))
return neighbors
def print_board(self, reveal=False):
for y in range(self.height):
row = ""
for x in range(self.width):
if not reveal and self.game_over == False:
row += "X "
else:
row += str(self.board[y][x]) + " "
print(row)
def play(self):
while self.game_over == False:
self.print_board()
x = int(input("Enter x coordinate: "))
y = int(input("Enter y coordinate: "))
if self.board[y][x] == "*":
print("Game over!")
self.game_over = True
else:
self.reveal(x, y)
if self.check_win():
print("You win!")
self.game_over = True
def reveal(self, x, y):
if self.board[y][x] != 0:
return
neighbors = self.get_neighbors(x, y)
for nx, ny in neighbors:
if self.board[ny][nx] == "*":
continue
if self.board[ny][nx] != 0:
self.board[ny][nx] = str(self.board[ny][nx])
else:
self.board[ny][nx] = "/"
self.reveal(nx, ny)
def check_win(self):
for y in range(self.height):
for x in range(self.width):
if self.board[y][x] == 0:
return False
return True
# example usage
game = Minesweeper(8, 8, 10)
game.play()
```
该游戏使用二维列表来表示扫雷板,其中包含了地雷和数字。玩家可以选择坐标来揭示扫雷板的某个格子。如果揭示到地雷,游戏结束。否则,玩家将揭示该格子周围的数字或空白格子。当所有非地雷格子都被揭示时,玩家获胜。
相关推荐
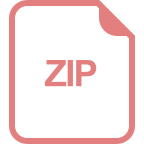
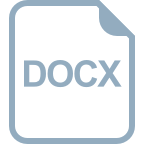







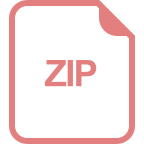
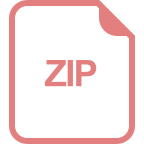
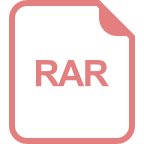