tkinter.Button
时间: 2023-07-22 07:05:19 浏览: 50
在tkinter中,Button组件有一个选项叫做command,它可以让你指定当用户点击按钮时要运行的函数。要使用command选项,你可以在Button创建时将其设置为相应的函数。例如,以下是一个创建Button并将其command设置为一个名为click的函数的示例代码:
```
import tkinter as tk
def click():
print("Button clicked")
root = tk.Tk()
button = tk.Button(root, text="Click me", command=click)
button.pack()
root.mainloop()
```
在这个例子中,我们创建了一个Button,将其文本设置为“Click me”,并将其command设置为click函数。当用户点击按钮时,click函数会被调用,并输出“Button clicked”。
相关问题
tkinter.button中relief参数
relief参数指定按钮外观的边框类型。它可以设置为SUNKEN、RAISED、GROOVE、RIDGE、FLAT、(默认值为FLAT)。
- SUNKEN:按钮呈现凹陷的外观。
- RAISED:按钮呈现凸起的外观。
- GROOVE:按钮外周呈现凹陷的边框,文本呈现凸起的外观。
- RIDGE:按钮外周呈现凸起的边框,文本呈现凹陷的外观。
- FLAT:按钮呈现平的外观,没有任何边框或呈现平的矩形边框。
例如,以下代码创建一个凹陷的按钮:
```python
import tkinter as tk
root = tk.Tk()
button = tk.Button(root, text="Click me!", relief=tk.SUNKEN)
button.pack()
root.mainloop()
```
import tkinter import tkinter.messagebox def Button_Click(btn): btn_Label = tkinter.Entry(mainUI) btn_Label.insert(tkinter.END,btn) btn_Label.place(x=45,y=75,width=40,height=20) mainUI = tkinter.Tk() mainUI.title('计算器') mainUI.geometry('300x280') #输入数字的两个文本框 num1_Label = tkinter.Label(mainUI,text='number 1 :',justify=tkinter.LEFT,anchor='e') num1_Label.place(x=25,y=20,width=80,height=30) num2_Label = tkinter.Label(mainUI,text='number 2 :',justify=tkinter.LEFT,anchor='e') num2_Label.place(x=25,y=125,width=80,height=30) num1_Var = tkinter.StringVar(mainUI,value='') num2_Var = tkinter.StringVar(mainUI,value='') num1_Entry = tkinter.Entry(mainUI,width=100,textvariable=num1_Var) num1_Entry.place(x=110,y=25,width=140,height=20) num2_Entry = tkinter.Entry(mainUI,width=100,textvariable=num2_Var) num2_Entry.place(x=110,y=130,width=140,height=20) #创建加减乘除按钮及显示文本框 sum_Button = tkinter.Button(mainUI,text='+',command=lambda :Button_Click('+')) sum_Button.place(x=100,y=75,width=30,height=20) min_Button = tkinter.Button(mainUI,text='-',command=lambda :Button_Click('-')) min_Button.place(x=145,y=75,width=30,height=20) mul_Button = tkinter.Button(mainUI,text='x',command=lambda :Button_Click('x')) mul_Button.place(x=190,y=75,width=30,height=20) div_Button = tkinter.Button(mainUI,text='/',command=lambda :Button_Click('/')) div_Button.place(x=235,y=75,width=30,height=20) #创建结果框以及计算按钮 cal_Label = tkinter.Label(mainUI,text='result :',justify=tkinter.LEFT,anchor='e') cal_Label.place(x=25,y=175,width=80,height=20) cal_Entry = tkinter.Entry(mainUI) cal_Entry.place(x=110,y=175,width=80,height=20) cal_Button = tkinter.Button(mainUI,text='Calculate') cal_Button.place(x=110,y=225,width=80,height=20) mainUI.mainloop()完善此代码中Calculate按钮被按下后进行的计算及输出操作
可以在Calculate按钮的command中添加一个函数来实现计算和输出的操作,具体实现如下:
1. 定义一个名为calculate的函数,用于计算并输出结果。
2. 在calculate函数中,获取num1_Entry和num2_Entry中的数值,并将其转换为浮点数类型。
3. 根据按钮的Label(即加减乘除符号),对两个数值进行相应的运算,并将结果显示在cal_Entry中。
修改后的代码如下:
```
import tkinter
import tkinter.messagebox
def Button_Click(btn):
btn_Label = tkinter.Entry(mainUI)
btn_Label.insert(tkinter.END, btn)
btn_Label.place(x=45, y=75, width=40, height=20)
def calculate():
# 获取num1_Entry和num2_Entry中的数值,并将其转换为浮点数类型
num1 = float(num1_Entry.get())
num2 = float(num2_Entry.get())
# 获取按钮的Label,根据加减乘除符号进行相应的运算
operator = btn_Label.get()
if operator == '+':
result = num1 + num2
elif operator == '-':
result = num1 - num2
elif operator == 'x':
result = num1 * num2
elif operator == '/':
if num2 == 0:
tkinter.messagebox.showerror('Error', '除数不能为0!')
return
else:
result = num1 / num2
# 将结果显示在cal_Entry中
cal_Entry.delete(0, tkinter.END)
cal_Entry.insert(tkinter.END, str(result))
mainUI = tkinter.Tk()
mainUI.title('计算器')
mainUI.geometry('300x280')
# 输入数字的两个文本框
num1_Label = tkinter.Label(mainUI, text='number 1 :', justify=tkinter.LEFT, anchor='e')
num1_Label.place(x=25, y=20, width=80, height=30)
num2_Label = tkinter.Label(mainUI, text='number 2 :', justify=tkinter.LEFT, anchor='e')
num2_Label.place(x=25, y=125, width=80, height=30)
num1_Var = tkinter.StringVar(mainUI, value='')
num2_Var = tkinter.StringVar(mainUI, value='')
num1_Entry = tkinter.Entry(mainUI, width=100, textvariable=num1_Var)
num1_Entry.place(x=110, y=25, width=140, height=20)
num2_Entry = tkinter.Entry(mainUI, width=100, textvariable=num2_Var)
num2_Entry.place(x=110, y=130, width=140, height=20)
# 创建加减乘除按钮及显示文本框
sum_Button = tkinter.Button(mainUI, text='+', command=lambda: Button_Click('+'))
sum_Button.place(x=100, y=75, width=30, height=20)
min_Button = tkinter.Button(mainUI, text='-', command=lambda: Button_Click('-'))
min_Button.place(x=145, y=75, width=30, height=20)
mul_Button = tkinter.Button(mainUI, text='x', command=lambda: Button_Click('x'))
mul_Button.place(x=190, y=75, width=30, height=20)
div_Button = tkinter.Button(mainUI, text='/', command=lambda: Button_Click('/'))
div_Button.place(x=235, y=75, width=30, height=20)
# 创建结果框以及计算按钮
cal_Label = tkinter.Label(mainUI, text='result :', justify=tkinter.LEFT, anchor='e')
cal_Label.place(x=25, y=175, width=80, height=20)
cal_Entry = tkinter.Entry(mainUI)
cal_Entry.place(x=110, y=175, width=80, height=20)
cal_Button = tkinter.Button(mainUI, text='Calculate', command=calculate)
cal_Button.place(x=110, y=225, width=80, height=20)
mainUI.mainloop()
```
相关推荐
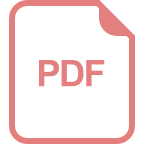
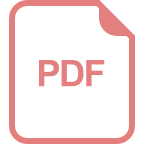
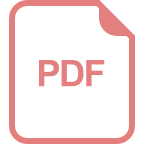













