java从标准中输入两组整数
时间: 2023-06-11 18:10:19 浏览: 134
您可以使用Java标准库中的Scanner类读取标准输入。以下是一个示例代码,它将读取两组整数并将它们存储在不同的变量中。
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 读取第一组整数
int a = scanner.nextInt();
int b = scanner.nextInt();
// 读取第二组整数
int c = scanner.nextInt();
int d = scanner.nextInt();
// 输出结果
System.out.println("第一组整数:" + a + " " + b);
System.out.println("第二组整数:" + c + " " + d);
}
}
```
当您运行这个程序时,您可以通过标准输入输入两组整数,如下所示:
```
1 2
3 4
```
程序将输出以下内容:
```
第一组整数:1 2
第二组整数:3 4
```
相关问题
java从标准中输入两组整数,求两组整数的异或集和交集,并按从大到小的顺序排列
以下是Java代码实现:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Set<Integer> set1 = new HashSet<>();
Set<Integer> set2 = new HashSet<>();
int n = sc.nextInt();
for (int i = 0; i < n; i++) {
set1.add(sc.nextInt());
}
int m = sc.nextInt();
for (int i = 0; i < m; i++) {
set2.add(sc.nextInt());
}
Set<Integer> intersection = new HashSet<>(set1);
intersection.retainAll(set2);
Set<Integer> xor = new HashSet<>(set1);
xor.addAll(set2);
xor.removeAll(intersection);
List<Integer> sorted = new ArrayList<>(xor);
Collections.sort(sorted, Collections.reverseOrder());
for (int num : intersection) {
sorted.add(num);
}
Collections.sort(sorted, Collections.reverseOrder());
for (int num : sorted) {
System.out.print(num + " ");
}
}
}
```
首先使用`Scanner`类从标准中输入两组整数,并将它们分别存储在`set1`和`set2`中。然后使用`retainAll()`方法找到两个集合的交集,并使用`addAll()`和`removeAll()`方法找到两个集合的异或集。接着将异或集和交集合并成一个列表,并按从大到小的顺序进行排序。最后,遍历排序后的列表并输出结果。
java从标准中输入两组整数(每行不超过20个,每组元素不重复)求两组整数的异或集(合并两组整数,去掉两组整数中都出现的整数形成的集合)和交集,并按从大到小的顺序排列
以下是Java代码实现:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 读取第一组整数
Set<Integer> set1 = new HashSet<>();
String line1 = scanner.nextLine();
String[] nums1 = line1.split(" ");
for (String num : nums1) {
set1.add(Integer.parseInt(num));
}
// 读取第二组整数
Set<Integer> set2 = new HashSet<>();
String line2 = scanner.nextLine();
String[] nums2 = line2.split(" ");
for (String num : nums2) {
set2.add(Integer.parseInt(num));
}
// 求异或集
Set<Integer> xorSet = new HashSet<>();
xorSet.addAll(set1);
xorSet.addAll(set2);
Set<Integer> intersection = new HashSet<>(set1);
intersection.retainAll(set2);
xorSet.removeAll(intersection);
// 按从大到小排序
List<Integer> resultList = new ArrayList<>(xorSet);
Collections.sort(resultList, Collections.reverseOrder());
// 输出结果
System.out.println("异或集:");
for (int num : resultList) {
System.out.print(num + " ");
}
System.out.println();
System.out.println("交集:");
for (int num : intersection) {
System.out.print(num + " ");
}
System.out.println();
}
}
```
示例输入:
```
1 3 5 7 9 11
2 4 6 8 10
```
示例输出:
```
异或集:
11 10 9 8 7 6 5 4 3 2 1
交集:
```
解释:两组整数没有重复元素,因此交集为空集,异或集为两组整数合并后去掉交集中的元素。按从大到小排序后输出。
阅读全文
相关推荐
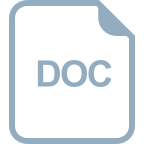
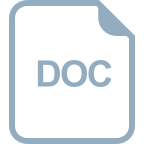
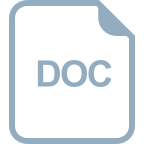



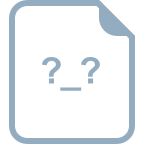









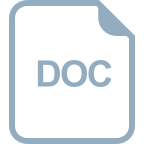