C语言线程池
时间: 2023-06-28 12:07:12 浏览: 66
线程池是一种常见的并发编程技术,它可以有效地管理线程,提高程序的并发性能。在 C 语言中,我们可以使用 POSIX 线程库来实现线程池。
以下是一个简单的 C 语言线程池实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 10
#define MAX_QUEUE 1000
typedef struct {
void (*function)(void *);
void *argument;
} task_t;
static pthread_mutex_t lock = PTHREAD_MUTEX_INITIALIZER;
static pthread_cond_t notify = PTHREAD_COND_INITIALIZER;
static task_t task_queue[MAX_QUEUE];
static int queue_head = 0, queue_tail = 0, queue_size = 0;
static int shutdown = 0;
static pthread_t thread_pool[MAX_THREADS];
static int thread_count = 0;
static void *thread_function(void *arg) {
while (1) {
pthread_mutex_lock(&lock);
while (queue_size == 0 && !shutdown) {
pthread_cond_wait(¬ify, &lock);
}
if (shutdown) {
pthread_mutex_unlock(&lock);
pthread_exit(NULL);
}
void (*task_function)(void *);
void *task_argument;
task_function = task_queue[queue_head].function;
task_argument = task_queue[queue_head].argument;
queue_size--;
queue_head++;
if (queue_head == MAX_QUEUE) {
queue_head = 0;
}
pthread_mutex_unlock(&lock);
(*task_function)(task_argument);
}
return NULL;
}
int thread_pool_init(int num_threads) {
if (num_threads <= 0 || num_threads > MAX_THREADS) {
num_threads = MAX_THREADS;
}
for (int i = 0; i < num_threads; i++) {
if (pthread_create(&thread_pool[i], NULL, thread_function, NULL) != 0) {
return -1;
}
thread_count++;
}
return 0;
}
int thread_pool_add_task(void (*function)(void *), void *argument) {
pthread_mutex_lock(&lock);
if (queue_size == MAX_QUEUE) {
pthread_mutex_unlock(&lock);
return -1;
}
task_queue[queue_tail].function = function;
task_queue[queue_tail].argument = argument;
queue_size++;
queue_tail++;
if (queue_tail == MAX_QUEUE) {
queue_tail = 0;
}
pthread_cond_signal(¬ify);
pthread_mutex_unlock(&lock);
return 0;
}
int thread_pool_destroy() {
pthread_mutex_lock(&lock);
shutdown = 1;
pthread_cond_broadcast(¬ify);
pthread_mutex_unlock(&lock);
for (int i = 0; i < thread_count; i++) {
pthread_join(thread_pool[i], NULL);
}
return 0;
}
```
使用方法如下:
```c
void print_message(void *arg) {
char *message = (char *) arg;
printf("%s\n", message);
free(message);
}
int main() {
thread_pool_init(4);
for (int i = 0; i < 8; i++) {
char *message = malloc(sizeof(char) * 20);
sprintf(message, "Task %d", i);
thread_pool_add_task(print_message, message);
}
thread_pool_destroy();
return 0;
}
```
该示例代码中,我们定义了一个任务结构体 `task_t`,包含了任务函数指针和参数。线程池中维护了一个任务队列,当有任务添加时,将任务加入队列,等待线程池中的线程来执行。线程池的每个线程都会从任务队列中取出一个任务并执行。线程池的销毁通过发送停止信号来实现,即将 `shutdown` 标志设置为 1,并唤醒所有等待条件变量的线程。最后,等待所有线程执行结束,然后退出线程池。
相关推荐
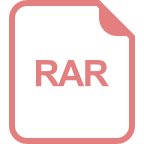













