class add(): def __init__(self,a,b): self.a=a self.b=b def add1(self): c=self.a+self.b print(f"{c}")
时间: 2024-05-14 21:19:17 浏览: 69
这是一个简单的 Python 类,名为 `add`,它有一个构造函数 `__init__`,接收两个参数 `a` 和 `b`。在构造函数中,它将参数 `a` 和 `b` 存储在实例变量 `self.a` 和 `self.b` 中。
类中还有一个方法 `add1`,它将实例变量 `self.a` 和 `self.b` 相加,并将结果存储在变量 `c` 中。最后,它使用 `print` 函数打印出 `c` 的值。
你可以使用以下代码创建一个 `add` 类的实例,并调用 `add1` 方法:
```python
example = add(2, 3)
example.add1()
```
这将输出 `5`。
相关问题
class Point: def __init__(self, x, y): self.__x = x self.__y = y def __add__(self, other): x = self.__x + other.__x y = self.__y + other.__y return Point(x, y) def __sub__(self, other): x = self.__x - other.__x y = self.__y - other.__y return Point(x, y) def __mul__(self, a): x = self.__x * a y = self.__y * a return Point(x, y) def __truediv__(self, a): x = self.__x / a y = self.__y / a return Point(x, y) r1 = Point(10, 20) r2 = Point(5, 5) print(r1+r2) print(r1-r2) print(r1*2) print(r1/2)代码改进,输出向量坐标
为了输出向量坐标,我们可以在 Point 类中添加一个方法来返回坐标的元组,如下所示:
```python
class Point:
def __init__(self, x, y):
self.__x = x
self.__y = y
def __add__(self, other):
x = self.__x + other.__x
y = self.__y + other.__y
return Point(x, y)
def __sub__(self, other):
x = self.__x - other.__x
y = self.__y - other.__y
return Point(x, y)
def __mul__(self, a):
x = self.__x * a
y = self.__y * a
return Point(x, y)
def __truediv__(self, a):
x = self.__x / a
y = self.__y / a
return Point(x, y)
def get_coordinates(self):
return (self.__x, self.__y)
```
然后我们就可以使用这个方法来输出向量坐标了,示例如下:
```python
r1 = Point(10, 20)
r2 = Point(5, 5)
print(r1+r2.get_coordinates()) # 输出 (15, 25)
print(r1-r2.get_coordinates()) # 输出 (5, 15)
print(r1*2).get_coordinates()) # 输出 (20, 40)
print(r1/2).get_coordinates()) # 输出 (5.0, 10.0)
```
class Node: def __init__(self, data=None, next=None): self.data = data self.next = nextclass LinkedList: def __init__(self): self.head = None def is_empty(self): return self.head is None def add(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node def length(self): count = 0 current = self.head while current is not None: count += 1 current = current.next return count def insert(self, data, position): if position == 0: self.add(data) else: new_node = Node(data) current = self.head for i in range(position - 1): current = current.next new_node.next = current.next current.next = new_node def remove(self, position): if position == 0: self.head = self.head.next else: current = self.head for i in range(position - 1): current = current.next current.next = current.next.next def traverse(self): current = self.head while current is not None: print(current.data) current = current.next
这是一个链表的实现,包括节点类 Node 和链表类 LinkedList。其中,节点类 Node 包含数据和指向下一个节点的指针,链表类 LinkedList 包含头节点和一些基本操作,如判断链表是否为空、添加节点、获取链表长度、在指定位置插入节点、删除指定位置的节点和遍历链表。
阅读全文
相关推荐
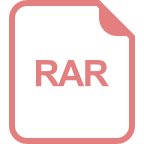
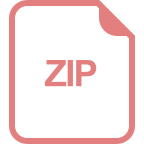
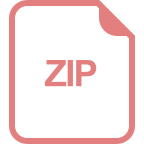














