本题要求实现两个函数,分别将读入的数据存储为单链表、将链表中所有存储了某给定值的结点删除。链表结点定义如下: struct listnode { int data; listnode *next; }; 函数接口定义: struct listnode *readlist(); struct listnode *deletem( struct listnode *l, int m ); 函数readlist从标准输入读入一系列正整数,按照读入顺序建立单链表。当读到−1时表示输入结束,函数应
时间: 2023-05-31 15:19:36 浏览: 274
### 回答1:
本题要求实现两个函数,分别将读入的数据存储为单链表、将链表中所有存储了某给定值的结点删除。链表结点定义如下:
struct listnode {
int data;
listnode *next;
};
函数接口定义如下:
struct listnode *readlist();
struct listnode *deletem( struct listnode *l, int m );
函数readlist从标准输入读入一个系列整数,按照输入顺序建立单链表。当读到-1时表示输入结束。函数返回指向链表第一个结点的指针。
函数deletem将链表l中所有存储了值为m的结点删除,并返回新的链表头结点指针。要求释放被删结点的空间。
注意:本题要求使用动态内存进行链表存储,当链表长度为0时,返回NULL指针。
### 回答2:
题目要求实现两个函数,分别是readlist和deletem。其中,readlist函数从标准输入读入一系列正整数,按照读入顺序建立单链表。当读到-1时表示输入结束。deletem函数将链表中所有存储了某给定值的结点删除。
首先,我们来看一下readlist函数的实现。该函数可以利用链表的插入操作,将读入的正整数插入到链表的尾部。当读到-1时,返回链表的头结点即可。
struct listnode *readlist()
{
int data;
struct listnode *head = NULL, *tail = NULL;
scanf("%d", &data);
while(data != -1)
{
struct listnode *node = malloc(sizeof(struct listnode));
node->data = data;
node->next = NULL;
if(head == NULL)
{
head = node;
tail = node;
}
else
{
tail->next = node;
tail = node;
}
scanf("%d", &data);
}
return head;
}
接着,我们来看一下deletem函数的实现。该函数需要遍历整个链表,找到存储了给定值的结点,并将其从链表中删除。可以利用链表的删除操作,同时需要保证被删除结点的前一个结点的next指针正确指向被删除结点的下一个结点。最后返回链表的头结点即可。
struct listnode *deletem(struct listnode *l, int m)
{
struct listnode *head = NULL, *tail = NULL;
while(l != NULL)
{
if(l->data != m)
{
struct listnode *node = malloc(sizeof(struct listnode));
node->data = l->data;
node->next = NULL;
if(head == NULL)
{
head = node;
tail = node;
}
else
{
tail->next = node;
tail = node;
}
}
l = l->next;
}
return head;
}
最后,我们可以将两个函数结合起来,实现完整的程序。
#include <stdio.h>
#include <stdlib.h>
struct listnode {
int data;
struct listnode *next;
};
struct listnode *readlist()
{
int data;
struct listnode *head = NULL, *tail = NULL;
scanf("%d", &data);
while(data != -1)
{
struct listnode *node = malloc(sizeof(struct listnode));
node->data = data;
node->next = NULL;
if(head == NULL)
{
head = node;
tail = node;
}
else
{
tail->next = node;
tail = node;
}
scanf("%d", &data);
}
return head;
}
struct listnode *deletem(struct listnode *l, int m)
{
struct listnode *head = NULL, *tail = NULL;
while(l != NULL)
{
if(l->data != m)
{
struct listnode *node = malloc(sizeof(struct listnode));
node->data = l->data;
node->next = NULL;
if(head == NULL)
{
head = node;
tail = node;
}
else
{
tail->next = node;
tail = node;
}
}
l = l->next;
}
return head;
}
int main()
{
struct listnode *head = readlist();
int m;
scanf("%d", &m);
struct listnode *new_head = deletem(head, m);
while(new_head != NULL)
{
printf("%d ", new_head->data);
new_head = new_head->next;
}
printf("\n");
return 0;
}
### 回答3:
题目要求我们实现两个函数,首先需要了解函数的功能和输入输出,才能成为功能完整的程序。
readlist函数
该函数的功能是从标准输入读入一系列正整数,按照读入顺序建立单链表。当读到-1时表示输入结束,函数应当返回链表头指针。
函数接口定义: struct listnode *readlist();
举例说明:假设输入的是6 3 1 4 8 -1,那么函数的输出应该是一个链表,其结构如下:
6 -> 3 -> 1 -> 4 -> 8 -> NULL
deletem函数
该函数的功能是将链表中所有存储了某给定值m的结点删除,最终返回删除后的链表。
函数接口定义: struct listnode *deletem( struct listnode *l, int m );
举例说明:假设链表为6 -> 3 -> 1 -> 4 -> 8 -> NULL,m的值为3,那么函数的输出应该是以下链表:
6 -> 1 -> 4 -> 8 -> NULL
具体实现
readlist函数的实现
在 readlist 函数中,我们需要按照以下步骤创建链表:
1. 创建头结点 head,指向 NULL。
该头结点仅用于存储链表的头指针。因为头结点不存放数据,所以 data 可以随便设定。
2. 创建指针 p 指向头结点,用于遍历链表;
3. 读入第一个数,创建第一个结点,并将头指针指向第一个结点;
4. 循环读入数据,每读入一个数据就创建一个结点,将 p 指向该结点;
5. 如果读入的数据是 -1,结束读数;否则继续执行第四步。
最后返回链表头指针。
代码如下:
struct listnode *readlist()
{
listnode *head = new listnode;
head->next = NULL;
listnode *p = head;
int data;
cin >> data;
while(data != -1)
{
listnode *node = new listnode;
node->data = data;
node->next = NULL;
p->next = node;
p = node;
cin >> data;
}
return head->next;
}
deletem函数的实现
在 deletem 函数中,我们需要按照以下步骤删除链表中值为 m 的结点:
1. 创建头结点 head,指向 NULL;
2. 设置指针 p 指向头结点;
3. 遍历链表,如果结点的 data 等于 m,则删除该结点;
4. 遍历结束后,返回链表头指针。
具体实现时,为了方便删除结点,我们需要在链表头结点后插入一个哨兵结点。
代码如下:
struct listnode *deletem( struct listnode *l, int m )
{
listnode *head = new listnode;
head->next = l;
listnode *p = head;
while(p->next != NULL)
{
if(p->next->data == m)
{
listnode *node = p->next;
p->next = node->next;
delete node;
}
else
{
p = p->next;
}
}
return head->next;
}
阅读全文
相关推荐







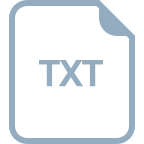
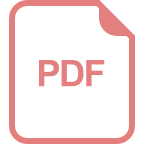
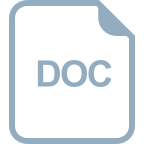
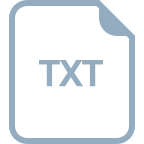



