编写一个基于C语言的传输1280字节数据的mqueue例程
时间: 2024-03-09 22:47:28 浏览: 75
以下是一个基于C语言的传输1280字节数据的mqueue例程,其中包括了消息队列的创建、发送和接收:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#define QUEUE_NAME "/my_queue"
#define MAX_MSG_SIZE 1280
#define MSG_NUM 10
int main() {
mqd_t mq;
struct mq_attr attr;
char buffer[MAX_MSG_SIZE];
int msg_prio = 1;
int i;
//设置消息队列属性
attr.mq_flags = 0;
attr.mq_maxmsg = MSG_NUM;
attr.mq_msgsize = MAX_MSG_SIZE;
attr.mq_curmsgs = 0;
//创建消息队列
mq_unlink(QUEUE_NAME);
mq = mq_open(QUEUE_NAME, O_CREAT | O_RDWR, 0666, &attr);
if (mq == -1) {
perror("mq_open");
exit(1);
}
//发送消息
for (i = 0; i < MSG_NUM; i++) {
sprintf(buffer, "This is message %d", i);
if (mq_send(mq, buffer, MAX_MSG_SIZE, msg_prio) == -1) {
perror("mq_send");
exit(1);
}
printf("Sent message %d\n", i);
}
//接收消息
for (i = 0; i < MSG_NUM; i++) {
if (mq_receive(mq, buffer, MAX_MSG_SIZE, &msg_prio) == -1) {
perror("mq_receive");
exit(1);
}
printf("Received message %d: %s\n", i, buffer);
}
//关闭消息队列
mq_close(mq);
mq_unlink(QUEUE_NAME);
return 0;
}
```
在这个例程中,我们首先设置了消息队列的属性,然后创建了消息队列。接着,我们通过循环发送了10条消息,每条消息的大小为1280字节。最后,我们又通过循环接收了这10条消息,并打印出了消息内容。最后,我们关闭了消息队列并删除了它。
阅读全文
相关推荐
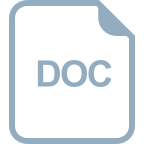
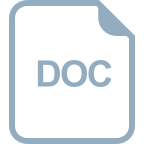
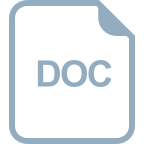


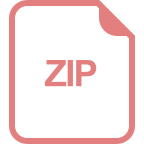
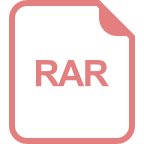
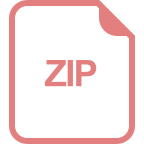
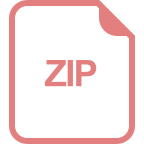
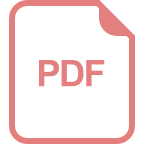
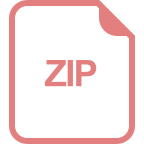
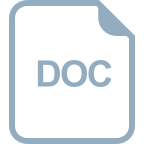






