int led_number=0; int led_number1=0; int led_number2=8; int r1=0; int r2=0;
时间: 2024-04-11 07:29:24 浏览: 8
您好!对于您提供的代码片段,我可以看出您定义了一些变量,并为它们赋予了一些初始值。其中包括:
- `led_number`:一个整数变量,初始值为0。
- `led_number1`:一个整数变量,初始值为0。
- `led_number2`:一个整数变量,初始值为8。
- `r1`:一个整数变量,初始值为0。
- `r2`:一个整数变量,初始值为0。
请问还有其他问题吗?
相关问题
#!/usr/bin/env python3 import os from Crypto.Util.number import getPrime, getRandomRange def isSquare(a, p): return pow(a, (p-1)//2, p) != p-1 class SquareRNG(object): def __init__(self, p, sa, sb): assert sa != 0 and sb != 0 (self.p, self.sa, self.sb) = (p, sa, sb) self.x = 0 def int(self, nbits): v, s = 0, 1 for _ in range(nbits): self.x = (self.x + 1) % p s += pow(self.sa, self.x, self.p) * pow(self.sb, self.x, self.p) s %= self.p v = (v << 1) | int(isSquare(s, self.p)) return v def bool(self): self.x = (self.x + 1) % self.p t = (pow(self.sa, self.x, self.p) + pow(self.sb, self.x, self.p)) t %= self.p return isSquare(t, self.p) p = getPrime(256) sb1 = int(input("Bob's seed 1: ")) % p sb2 = int(input("Bob's seed 2: ")) % p for _ in range(77): sa = getRandomRange(1, p) r1 = SquareRNG(p, sa, sb1) print("Random 1:", hex(r1.int(32))) r2 = SquareRNG(p, sa, sb2) print("Random 2:", hex(r2.int(32))) guess = int(input("Guess next bool [0 or 1]: ")) if guess == int(r1.bool()): print("OK!") else: print("NG...") break else: print("Congratz!") print(os.getenv("FLAG", "nek0pts{*** REDACTED ***}"))
以下是您提供的Python代码的翻译版本:
```python
#!/usr/bin/env python3
import os
from Crypto.Util.number import getPrime, getRandomRange
def isSquare(a, p):
return pow(a, (p-1)//2, p) != p-1
class SquareRNG(object):
def __init__(self, p, sa, sb):
assert sa != 0 and sb != 0
(self.p, self.sa, self.sb) = (p, sa, sb)
self.x = 0
def int(self, nbits):
v, s = 0, 1
for _ in range(nbits):
self.x = (self.x + 1) % p
s += pow(self.sa, self.x, self.p) * pow(self.sb, self.x, self.p)
s %= self.p
v = (v << 1) | int(isSquare(s, self.p))
return v
def bool(self):
self.x = (self.x + 1) % self.p
t = (pow(self.sa, self.x, self.p) + pow(self.sb, self.x, self.p))
t %= self.p
return isSquare(t, self.p)
p = getPrime(256)
sb1 = int(input("Bob's seed 1: ")) % p
sb2 = int(input("Bob's seed 2: ")) % p
for _ in range(77):
sa = getRandomRange(1, p)
r1 = SquareRNG(p, sa, sb1)
print("Random 1:", hex(r1.int(32)))
r2 = SquareRNG(p, sa, sb2)
print("Random 2:", hex(r2.int(32)))
guess = int(input("Guess next bool [0 or 1]: "))
if guess == int(r1.bool()):
print("OK!")
else:
print("NG...")
break
else:
print("Congratz!")
print(os.getenv("FLAG", "nek0pts{*** REDACTED ***}"))
```
请注意,这是直接的翻译,可能需要根据您的具体需求进行进一步调整和优化。如果您有任何其他问题,请随时提问。
RationalNumber类C++
下面是一个简单的实现RationalNumber类的C++代码:
```c++
#include <iostream>
using namespace std;
class RationalNumber {
private:
int numerator; // 分子
int denominator; // 分母
public:
RationalNumber() {
numerator = 0;
denominator = 1;
}
RationalNumber(int numerator, int denominator) {
this->numerator = numerator;
this->denominator = denominator;
simplify(); // 约分
}
// 获取分子
int getNumerator() const {
return numerator;
}
// 获取分母
int getDenominator() const {
return denominator;
}
// 赋值运算符重载
RationalNumber& operator=(const RationalNumber& other) {
if (this != &other) {
numerator = other.numerator;
denominator = other.denominator;
}
return *this;
}
// 加法运算符重载
RationalNumber operator+(const RationalNumber& other) {
int newNumerator = numerator * other.denominator + other.numerator * denominator;
int newDenominator = denominator * other.denominator;
return RationalNumber(newNumerator, newDenominator);
}
// 减法运算符重载
RationalNumber operator-(const RationalNumber& other) {
int newNumerator = numerator * other.denominator - other.numerator * denominator;
int newDenominator = denominator * other.denominator;
return RationalNumber(newNumerator, newDenominator);
}
// 乘法运算符重载
RationalNumber operator*(const RationalNumber& other) {
int newNumerator = numerator * other.numerator;
int newDenominator = denominator * other.denominator;
return RationalNumber(newNumerator, newDenominator);
}
// 除法运算符重载
RationalNumber operator/(const RationalNumber& other) {
int newNumerator = numerator * other.denominator;
int newDenominator = denominator * other.numerator;
return RationalNumber(newNumerator, newDenominator);
}
// 约分
void simplify() {
int gcd = getGCD(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
if (denominator < 0) {
numerator *= -1;
denominator *= -1;
}
}
// 获取最大公约数
int getGCD(int a, int b) {
if (b == 0) {
return a;
}
return getGCD(b, a % b);
}
// 输出有理数
void print() const {
if (denominator == 1) {
cout << numerator << endl;
} else {
cout << numerator << "/" << denominator << endl;
}
}
};
int main() {
RationalNumber r1(2, 3);
RationalNumber r2(3, 4);
RationalNumber r3 = r1 + r2;
RationalNumber r4 = r1 - r2;
RationalNumber r5 = r1 * r2;
RationalNumber r6 = r1 / r2;
cout << "r1 = ";
r1.print();
cout << "r2 = ";
r2.print();
cout << "r1 + r2 = ";
r3.print();
cout << "r1 - r2 = ";
r4.print();
cout << "r1 * r2 = ";
r5.print();
cout << "r1 / r2 = ";
r6.print();
return 0;
}
```
在该代码中,定义了一个 `RationalNumber` 类,包含了分子和分母两个私有成员变量,以及一些公有成员函数,包括构造函数、赋值运算符重载、加法、减法、乘法、除法运算符重载、约分、获取最大公约数、输出有理数等。在 `main` 函数中,创建了两个有理数对象 `r1` 和 `r2`,并对其进行了加、减、乘、除等运算,最后输出了结果。
相关推荐
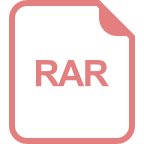







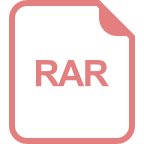
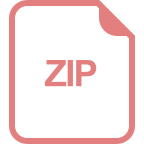
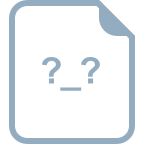