python 查找dataframe中元素位置
时间: 2023-09-24 10:11:21 浏览: 146
你可以使用 `pandas.DataFrame.where()` 方法来查找DataFrame中元素的位置。下面是一个例子:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': ['a', 'b', 'c']})
# 查找值为2的元素的位置
indices = df.where(df == 2).stack().index.tolist()
print(indices)
```
输出:
```
[(0, 'A')]
```
这表示值为2的元素在第0行第A列。如果要查找多个元素的位置,只需在 `where()` 方法中使用逻辑运算符,例如:
```python
# 查找值为2或3的元素的位置
indices = df.where((df == 2) | (df == 3)).stack().index.tolist()
print(indices)
```
输出:
```
[(0, 'A'), (1, 'A')]
```
这表示值为2的元素在第0行第A列,值为3的元素在第1行第A列。
相关问题
dataframe 查找数字位置
如果你想查找一个 DataFrame 中某个数值的位置,可以使用 `numpy` 库中的 `where()` 函数。假设你的 DataFrame 名称为 `df`,你想查找数值为 `x` 的位置,可以使用以下代码:
```python
import numpy as np
# 查找数值为 x 的位置
rows, cols = np.where(df.values == x)
# 打印位置
for row, col in zip(rows, cols):
print('({},{})'.format(row, col))
```
其中,`rows` 和 `cols` 分别是一个一维数组,表示数值为 `x` 的位置的行号和列号。如果有多个数值为 `x`,那么 `rows` 和 `cols` 数组会有多个元素,你可以使用 `zip()` 函数来遍历这些位置并进行处理。
如何查找dataframe一列中是否存在重复的元素
你可以使用 Pandas 库中的 `duplicated` 方法来查找一列中是否存在重复的元素。下面是示例代码:
``` python
import pandas as pd
# 创建一个包含重复元素的 DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 3, 4, 5]})
# 查找是否存在重复元素
duplicated = df['A'].duplicated()
# 打印结果
print(duplicated)
```
输出结果为:
```
0 False
1 False
2 False
3 True
4 False
5 False
Name: A, dtype: bool
```
其中,`duplicated` 返回一个布尔型的 Series,表示每个元素是否为重复元素。在上述示例中,第 4 行和第 5 行的元素是重复的,因此对应位置的值为 `True`。
阅读全文
相关推荐
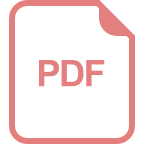
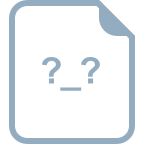
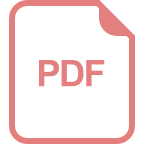
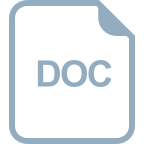
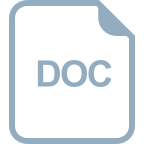
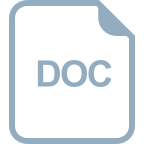
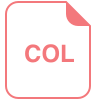
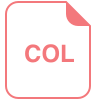
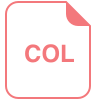
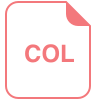
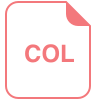




