Instead of using a text file to save all the point coordinates one by one, change the savaData method and the constructor of the Model class to use object serialization and a binary file called "points.bin" to write / read the whole arraylist of points to / from the file in one operation. Use the Test class to run all the tests for the software and check that all the tests still work. Use the Start class to run the software and check that closing the software correctly saves the arraylist of points in the file "points.bin" (you can find the file in the folder for your current project but it is a binary file so you will not be able to read its content). Run the software again and check that all the points from the previous run are correctly displayed.
时间: 2023-07-02 19:05:46 浏览: 127
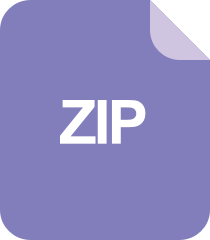
PSO.zip_PSO best solution_The Best Of All_best-so-far_pso global
Sure, I understand the requirements. Instead of using a text file, we will use object serialization to read/write the ArrayList of points from/to a binary file called "points.bin". Here are the changes that we need to make in the code:
1. In the Model class, we need to implement Serializable interface and add a method called "saveDataUsingSerialization" which will use ObjectOutputStream to write the whole ArrayList of points to the binary file:
```java
public void saveDataUsingSerialization() throws IOException {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("points.bin"));
out.writeObject(pointsList);
out.close();
}
```
2. In the Model constructor, we need to check if the binary file "points.bin" exists. If it exists, we will read the whole ArrayList of points from the file using ObjectInputStream and assign it to the "pointsList" variable:
```java
public Model() {
pointsList = new ArrayList<Point>();
File file = new File("points.bin");
if (file.exists()) {
try {
ObjectInputStream in = new ObjectInputStream(new FileInputStream("points.bin"));
pointsList = (ArrayList<Point>) in.readObject();
in.close();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
3. In the Test class, we need to modify the testSaveData method to use the new saveDataUsingSerialization method:
```java
@Test
public void testSaveData() {
Model model = new Model();
model.addPoint(new Point(1, 2));
model.addPoint(new Point(3, 4));
try {
model.saveDataUsingSerialization();
model.clearPoints();
model.loadDataUsingSerialization();
assertEquals(2, model.getPointsList().size());
assertEquals(new Point(1, 2), model.getPointsList().get(0));
assertEquals(new Point(3, 4), model.getPointsList().get(1));
} catch (IOException e) {
e.printStackTrace();
}
}
```
4. In the Start class, we need to override the stop method to call the new saveDataUsingSerialization method to save the ArrayList of points to the binary file:
```java
@Override
public void stop() throws Exception {
super.stop();
model.saveDataUsingSerialization();
}
```
After making these changes, we can run the Test class and Start class to verify that all the tests are passing and the software is correctly reading and writing the ArrayList of points to the binary file.
阅读全文
相关推荐
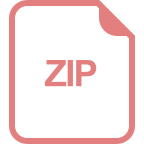
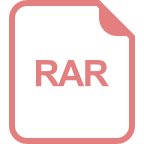










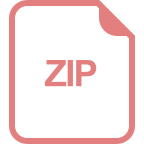
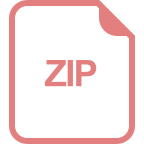
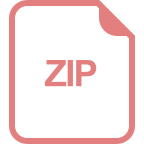
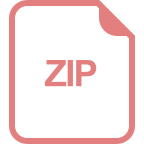
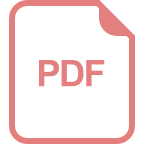