import pygame import time import random pygame.init() screen = pygame.display.set_mode((600, 500)) pygame.display.set_caption("接小球游戏") ball_x = 300 ball_y = 250 rect_x, rect_y, rect_w, rect_h = 300, 460, 120, 40 font1 = pygame.font.Font(None, 24) score = 0 lives = 3 def ball(ball_x, ball_y): pygame.draw.circle(screen, (255., 25, 52), (ball_x, ball_y), 20, 0) while True: for event in pygame.event.get(): print(event) if event.type == pygame.QUIT: pygame.quit() elif event.type == pygame.MOUSEMOTION: rect_x, _ = event.pos screen.fill((34, 177, 135)) ball_y = ball_y + 1 if ball_y > 500: ball_y = 0 ball_x = random.randint(0, 600) lives = lives -1 ball(ball_x, ball_y) if rect_x < ball_x < rect_x + rect_w and rect_y < ball_y < rect_y + rect_h: score = score + 1 ball_y = 0 ball_x = random.randint(0, 600) Text_score = font1.render('score:%d' % score, True, (0, 0, 0)) screen.blit(Text_score, (0, 0)) Text_lives = font1.render('lives:%d' % lives, True, (0, 0, 0)) screen.blit(Text_lives, (530, 0)) pygame.draw.rect(screen, (100, 200, 30), (rect_x, rect_y, rect_w, rect_h), 0) pygame.display.update() pygame.quit() 把这个代码完善升级,并解释升级后每行代码含义
时间: 2023-06-14 11:07:43 浏览: 75
import pygame # 导入pygame库
import time # 导入time库
import random # 导入random库
pygame.init() # 初始化pygame
screen = pygame.display.set_mode((600, 500)) # 创建窗口
pygame.display.set_caption("接小球游戏") # 设置窗口标题
# 初始化小球和矩形框的位置和大小
ball_x = 300
ball_y = 250
rect_x, rect_y, rect_w, rect_h = 300, 460, 120, 40
font1 = pygame.font.Font(None, 24) # 创建字体对象
score = 0 # 记录得分
lives = 3 # 记录生命值
# 定义绘制小球的函数
def ball(ball_x, ball_y):
pygame.draw.circle(screen, (255., 25, 52), (ball_x, ball_y), 20, 0)
while True:
for event in pygame.event.get(): # 处理每一个事件
print(event) # 打印事件
if event.type == pygame.QUIT: # 判断是否退出游戏
pygame.quit() # 退出pygame
elif event.type == pygame.MOUSEMOTION: # 处理鼠标移动事件
rect_x, _ = event.pos # 更新矩形框的位置
screen.fill((34, 177, 135)) # 填充背景色
ball_y = ball_y + 1 # 更新小球的位置
if ball_y > 500: # 判断小球是否出界
ball_y = 0
ball_x = random.randint(0, 600)
lives = lives -1 # 生命值减1
ball(ball_x, ball_y) # 绘制小球
if rect_x < ball_x < rect_x + rect_w and rect_y < ball_y < rect_y + rect_h: # 判断是否接到小球
score = score + 1
ball_y = 0
ball_x = random.randint(0, 600)
Text_score = font1.render('score:%d' % score, True, (0, 0, 0)) # 创建得分文本对象
screen.blit(Text_score, (0, 0)) # 绘制得分文本
Text_lives = font1.render('lives:%d' % lives, True, (0, 0, 0)) # 创建生命值文本对象
screen.blit(Text_lives, (530, 0)) # 绘制生命值文本
pygame.draw.rect(screen, (100, 200, 30), (rect_x, rect_y, rect_w, rect_h), 0) # 绘制矩形框
pygame.display.update() # 更新屏幕
pygame.quit() # 退出pygame
本代码升级了原来的“接小球游戏”,新增了生命值、得分、矩形框等元素。
含义如下:
1. 导入pygame、time和random库;
2. 初始化pygame;
3. 创建窗口;
4. 初始化小球和矩形框的位置和大小;
5. 创建字体对象;
6. 记录得分和生命值;
7. 定义绘制小球的函数;
8. 循环处理每一个事件,包括退出游戏、鼠标移动等事件;
9. 填充背景色;
10. 更新小球的位置;
11. 判断小球是否出界,如果出界,更新小球位置并减少生命值;
12. 绘制小球;
13. 判断是否接到小球,如果接到,增加得分;
14. 创建得分文本对象,绘制得分文本;
15. 创建生命值文本对象,绘制生命值文本;
16. 绘制矩形框;
17. 更新屏幕;
18. 退出pygame。
阅读全文
相关推荐
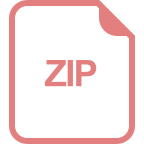
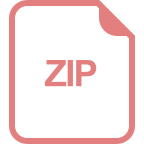













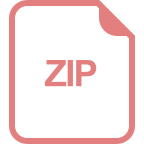