用java写一个打地鼠游戏
时间: 2023-12-22 07:03:34 浏览: 19
以下是一个简单的打地鼠游戏Java实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.Random;
public class WhackAMole extends JFrame implements ActionListener {
private final int ROWS = 5;
private final int COLS = 5;
private final int NUM_MOLES = 10;
private final int TIME_LIMIT = 30;
private JButton[][] buttons;
private Timer timer;
private JLabel scoreLabel;
private int score;
private int molesLeft;
private int timeLeft;
public WhackAMole() {
super("Whack-A-Mole");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(ROWS, COLS));
buttons = new JButton[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
buttons[i][j] = new JButton("");
buttons[i][j].setActionCommand(i + " " + j);
buttons[i][j].addActionListener(this);
buttonPanel.add(buttons[i][j]);
}
}
add(buttonPanel, BorderLayout.CENTER);
JPanel infoPanel = new JPanel();
infoPanel.setLayout(new GridLayout(1, 2));
scoreLabel = new JLabel("Score: 0");
infoPanel.add(scoreLabel);
JLabel timeLabel = new JLabel("Time Left: " + TIME_LIMIT);
infoPanel.add(timeLabel);
add(infoPanel, BorderLayout.SOUTH);
pack();
setLocationRelativeTo(null);
setVisible(true);
timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
timeLeft--;
timeLabel.setText("Time Left: " + timeLeft);
if (timeLeft == 0) {
timer.stop();
JOptionPane.showMessageDialog(WhackAMole.this, "Game over! Your score is " + score);
}
}
});
startGame();
}
private void startGame() {
score = 0;
molesLeft = NUM_MOLES;
timeLeft = TIME_LIMIT;
scoreLabel.setText("Score: " + score);
timer.restart();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
buttons[i][j].setEnabled(true);
buttons[i][j].setText("");
}
}
Random rand = new Random();
for (int i = 0; i < NUM_MOLES; i++) {
int row, col;
do {
row = rand.nextInt(ROWS);
col = rand.nextInt(COLS);
} while (!buttons[row][col].getText().equals(""));
buttons[row][col].setText("M");
}
}
@Override
public void actionPerformed(ActionEvent e) {
String[] tokens = e.getActionCommand().split(" ");
int row = Integer.parseInt(tokens[0]);
int col = Integer.parseInt(tokens[1]);
if (buttons[row][col].getText().equals("M")) {
buttons[row][col].setText("");
score++;
molesLeft--;
scoreLabel.setText("Score: " + score);
if (molesLeft == 0) {
timer.stop();
JOptionPane.showMessageDialog(this, "You win! Your score is " + score);
}
} else {
score--;
scoreLabel.setText("Score: " + score);
}
}
public static void main(String[] args) {
new WhackAMole();
}
}
```
这个游戏界面由一个5x5的按钮网格和一个显示得分和剩余时间的信息面板组成。游戏开始后,随机生成10只地鼠,每只地鼠随机出现在一个按钮上。玩家需要尽可能快地点击出现的地鼠,每击中一只得1分,错过一只扣1分。游戏时间为30秒,时间到后游戏结束,得分高者获胜。
相关推荐
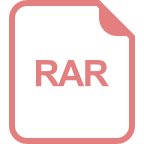
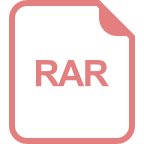














