dataframe按默认索引排序
时间: 2023-07-21 16:30:55 浏览: 76
可以使用`sort_index()`方法按照默认索引排序,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 4, 2], 'B': [3, 1, 2]})
print(df)
# Output:
# A B
# 0 1 3
# 1 4 1
# 2 2 2
sorted_df = df.sort_index()
print(sorted_df)
# Output:
# A B
# 0 1 3
# 1 4 1
# 2 2 2
```
默认情况下,`sort_index()`方法会按照行索引进行排序。如果要按照列索引排序,可以使用`axis`参数指定为`1`,例如:
```python
sorted_df = df.sort_index(axis=1)
print(sorted_df)
# Output:
# A B
# 0 1 3
# 1 4 1
# 2 2 2
```
这样就按照列索引排序了。
相关问题
dataframe按照索引列排序
可以使用`sort_index`方法按照索引列排序。下面是一个例子:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 32, 18, 47],
'city': ['New York', 'Paris', 'London', 'Tokyo']})
# 按照索引列排序
df_sorted = df.sort_index()
print(df_sorted)
```
输出结果如下:
```
name age city
0 Alice 25 New York
1 Bob 32 Paris
2 Charlie 18 London
3 David 47 Tokyo
```
默认情况下,`sort_index`方法会按照升序排序。如果需要降序排序,可以设置参数`ascending=False`,例如:
```python
df_sorted = df.sort_index(ascending=False)
```
这样就会按照降序排序。
sort_index()方法可以将Series或DataFrame对象按值排序
这个说法是不完全正确的。`sort_index()`方法可以对`Series`或`DataFrame`对象按照索引进行排序,而不是按照值进行排序。如果想要按照值对`Series`或`DataFrame`对象进行排序,应该使用`sort_values()`方法,该方法可以按照指定轴上的值进行排序。需要注意的是,`sort_values()`方法默认是升序排序,如果需要降序排序,可以设置`ascending=False`参数。
阅读全文
相关推荐
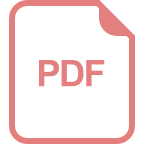

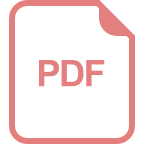











