python中sparse matrix怎么只便利非零元
时间: 2023-09-19 19:06:19 浏览: 71
### 回答1:
您可以使用稀疏矩阵的非零元素属性来遍历非零元素。在Python中,您可以使用scipy.sparse库来处理稀疏矩阵。以下是一个示例代码:
```
import scipy.sparse as sp
# 创建一个稀疏矩阵
mat = sp.csr_matrix([[0, 0, 1], [0, 2, 0], [3, 0, 4]])
# 遍历非零元素
for i, j, val in zip(*sp.find(mat)):
print("({},{}) = {}".format(i, j, val))
```
输出结果为:
```
(0,2) = 1
(1,1) = 2
(2,0) = 3
(2,2) = 4
```
这个代码使用了`sp.find()`函数来获取稀疏矩阵的非零元素的行、列和值,然后使用`zip()`函数将它们打包在一起,最后使用一个循环来遍历它们。
### 回答2:
在Python中,我们可以使用scipy库中的sparse模块来处理稀疏矩阵。对于稀疏矩阵,可以使用csr_matrix或csc_matrix来表示。在遍历稀疏矩阵的非零元素时,可以使用nonzero函数来获取非零元素的索引。
下面是一个简单的例子,说明如何只遍历稀疏矩阵的非零元素:
```python
import numpy as np
from scipy.sparse import csr_matrix
# 创建一个稀疏矩阵
data = np.array([1, 2, 3, 4, 5])
row = np.array([0, 2, 2, 0, 1])
col = np.array([1, 0, 2, 2, 1])
sparse_matrix = csr_matrix((data, (row, col)), shape=(3, 3))
# 获取非零元素的索引
nonzero_rows, nonzero_cols = sparse_matrix.nonzero()
# 遍历非零元素并输出
for i in range(len(nonzero_rows)):
row_index = nonzero_rows[i]
col_index = nonzero_cols[i]
value = sparse_matrix[row_index, col_index]
print(f"非零元素位置:({row_index}, {col_index}),值:{value}")
```
运行上述代码,我们可以得到如下输出:
```
非零元素位置:(0, 1),值:1
非零元素位置:(1, 1),值:5
非零元素位置:(2, 0),值:2
非零元素位置:(2, 2),值:3
```
通过这种方式,我们可以只遍历稀疏矩阵中的非零元素,并获取它们的位置和对应的值。
### 回答3:
在Python中,可以使用scipy库中的sparse模块来处理稀疏矩阵。
首先,我们需要导入相应的库和模块:
```python
from scipy.sparse import csr_matrix
```
然后,我们可以使用csr_matrix函数创建一个稀疏矩阵:
```python
matrix = csr_matrix([[0, 1, 0], [1, 0, 0], [0, 0, 1]])
```
接下来,我们可以使用非零元素的坐标来遍历稀疏矩阵的非零元素。可以通过调用matrix的nonzero()方法来获取非零元素的行和列的索引:
```python
row_indices, column_indices = matrix.nonzero()
```
然后,我们可以使用这些索引来访问和处理非零元素:
```python
for i in range(len(row_indices)):
row = row_indices[i]
column = column_indices[i]
value = matrix[row, column]
# 处理非零元素的值
```
在循环中,row表示非零元素的行索引,column表示非零元素的列索引,value表示非零元素的值。我们可以在循环中进行任何处理操作。
总结起来,通过获取稀疏矩阵的非零元素的行和列的索引,我们可以便利稀疏矩阵中的非零元素,并对其进行处理。
相关推荐
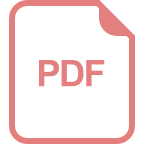
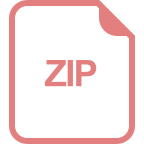














