请编写stm32f103与hc-05蓝牙与手机连接发送数据
时间: 2023-09-30 10:03:30 浏览: 55
好的,以下是一个示例代码,供您参考:
```c
#include "stm32f10x.h"
#include "stm32f10x_usart.h"
#include "stm32f10x_gpio.h"
/* 定义蓝牙串口 */
#define BLUETOOTH_USART USART1
/* 定义蓝牙串口的GPIO口 */
#define BLUETOOTH_USART_GPIO GPIOA
#define BLUETOOTH_USART_TX_PIN GPIO_Pin_9
#define BLUETOOTH_USART_RX_PIN GPIO_Pin_10
/* 定义蓝牙模块的状态 */
typedef enum {
BLUETOOTH_DISCONNECTED = 0,
BLUETOOTH_CONNECTED = 1
} BluetoothState;
/* 定义蓝牙模块的状态变量 */
static BluetoothState bluetoothState = BLUETOOTH_DISCONNECTED;
/* 定义发送数据的缓冲区 */
static uint8_t txBuffer[50];
/* 初始化蓝牙模块 */
void bluetoothInit(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
/* 使能GPIOA的时钟 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
/* 配置TX引脚为推挽输出 */
GPIO_InitStruct.GPIO_Pin = BLUETOOTH_USART_TX_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(BLUETOOTH_USART_GPIO, &GPIO_InitStruct);
/* 配置RX引脚为浮空输入 */
GPIO_InitStruct.GPIO_Pin = BLUETOOTH_USART_RX_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(BLUETOOTH_USART_GPIO, &GPIO_InitStruct);
/* 使能USART1的时钟 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
/* 配置USART1的参数 */
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(BLUETOOTH_USART, &USART_InitStruct);
/* 配置USART1的中断 */
NVIC_InitStruct.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
/* 使能USART1的接收中断 */
USART_ITConfig(BLUETOOTH_USART, USART_IT_RXNE, ENABLE);
/* 使能USART1 */
USART_Cmd(BLUETOOTH_USART, ENABLE);
}
/* 发送数据 */
void bluetoothSendData(uint8_t* data, uint32_t length)
{
uint32_t i;
/* 等待蓝牙模块连接 */
while (bluetoothState == BLUETOOTH_DISCONNECTED);
/* 将数据写入发送缓冲区 */
for (i = 0; i < length; i++) {
txBuffer[i] = data[i];
}
/* 发送数据 */
USART_SendData(BLUETOOTH_USART, txBuffer[0]);
}
/* USART1中断处理函数 */
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(BLUETOOTH_USART, USART_IT_RXNE) != RESET) {
uint8_t data = USART_ReceiveData(BLUETOOTH_USART);
/* 判断是否收到连接成功的消息 */
if (data == 'A') {
bluetoothState = BLUETOOTH_CONNECTED;
}
}
}
int main(void)
{
/* 初始化蓝牙模块 */
bluetoothInit();
while (1) {
/* 发送数据 */
bluetoothSendData("Hello, World!\r\n", 15);
/* 延时 */
for (int i = 0; i < 0x3FFFF; i++);
}
}
```
以上代码中,我们使用了STM32F103的USART1串口与HC-05蓝牙模块进行通信。在初始化蓝牙模块时,我们配置了USART1的参数,并使能了接收中断。在发送数据时,我们先等待蓝牙模块连接成功,然后将数据写入发送缓冲区,并调用USART_SendData函数发送数据。在接收到蓝牙模块发来的数据时,我们判断是否收到了连接成功的消息,并将状态变量bluetoothState设为BLUETOOTH_CONNECTED。在主函数中,我们不断发送数据,并进行一定的延时。
请注意,以上代码仅供参考,如需使用请根据实际情况进行适当的修改。
相关推荐
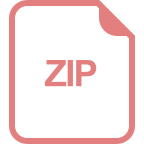
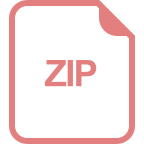














