java替换指定字符串
时间: 2023-06-07 20:10:09 浏览: 92
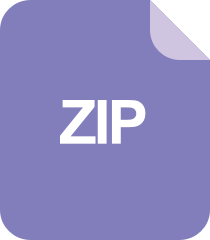
java 替换指定的字符
好的,关于Java替换指定字符串,你可以使用String类的replace方法。例如,如果你想把字符串s中所有的"cat"替换成"dog",可以这样做:
```
String s = "I have a cat. My cat is cute.";
String replaced = s.replace("cat", "dog");
System.out.println(replaced);
```
执行结果为:"I have a dog. My dog is cute."。
你可以将想要替换的字符串和替换后的字符串作为参数传递给replace方法,就可以完成字符串替换。
阅读全文
相关推荐















