在双向链表中插入一个结点
时间: 2024-05-03 09:12:54 浏览: 100
在双向链表中插入一个结点需要执行以下步骤:
1. 创建一个新的节点,将要插入的数据存储在该节点中。
2. 找到插入位置,即要插入节点的前一个节点和后一个节点。
3. 将前一个节点的next指针指向新节点,将后一个节点的prev指针指向新节点,这样新节点就被插入到了双向链表中。
4. 如果插入的是头节点,需要将链表的头指针指向新节点。
下面是一个示例代码:
```
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
void insertNode(struct Node** head, int data, int position) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
if(position == 1) {
newNode->next = *head;
(*head)->prev = newNode;
*head = newNode;
return;
}
struct Node* current = *head;
int i;
for(i = 1; i < position-1 && current != NULL; i++) {
current = current->next;
}
if(current == NULL) {
printf("Invalid position\n");
return;
}
newNode->next = current->next;
current->next = newNode;
newNode->prev = current;
if(newNode->next != NULL) {
newNode->next->prev = newNode;
}
}
```
阅读全文
相关推荐
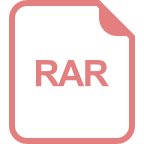
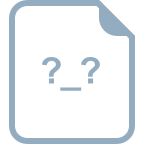
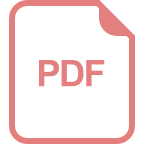















