提供一下springboot中ftp并行上传多个文件的代码
时间: 2024-03-02 22:49:21 浏览: 76
以下是基于Spring Boot的FTP并行上传多个文件的示例代码:
```
@Service
public class FtpService {
@Value("${ftp.host}")
private String ftpHost;
@Value("${ftp.port}")
private int ftpPort;
@Value("${ftp.username}")
private String ftpUsername;
@Value("${ftp.password}")
private String ftpPassword;
private FTPClient ftpClient;
/**
* 获取FTP连接
*
* @return FTPClient对象
* @throws IOException
*/
private FTPClient getFtpClient() throws IOException {
if (ftpClient == null) {
ftpClient = new FTPClient();
ftpClient.connect(ftpHost, ftpPort);
ftpClient.login(ftpUsername, ftpPassword);
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
ftpClient.enterLocalPassiveMode();
}
return ftpClient;
}
/**
* 并行上传多个文件
*
* @param fileMap 文件Map,key为文件名,value为文件流
* @param dirPath 上传目录路径
* @throws IOException
* @throws InterruptedException
*/
public void uploadFiles(Map<String, InputStream> fileMap, String dirPath) throws IOException, InterruptedException {
ExecutorService executorService = Executors.newFixedThreadPool(fileMap.size());
List<Future<Void>> futures = new ArrayList<>();
for (Map.Entry<String, InputStream> entry : fileMap.entrySet()) {
String fileName = entry.getKey();
InputStream inputStream = entry.getValue();
futures.add(executorService.submit(() -> {
uploadFile(inputStream, dirPath, fileName);
return null;
}));
}
for (Future<Void> future : futures) {
future.get();
}
executorService.shutdown();
}
/**
* 上传单个文件
*
* @param inputStream 文件流
* @param dirPath 上传目录路径
* @param fileName 文件名
* @throws IOException
*/
private void uploadFile(InputStream inputStream, String dirPath, String fileName) throws IOException {
FTPClient ftpClient = getFtpClient();
ftpClient.changeWorkingDirectory(dirPath);
ftpClient.enterLocalPassiveMode();
boolean success = ftpClient.storeFile(fileName, inputStream);
if (!success) {
throw new IOException("Failed to upload file: " + fileName);
}
}
/**
* 关闭FTP连接
*
* @throws IOException
*/
public void close() throws IOException {
if (ftpClient != null && ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
}
```
在上述代码中,`FtpService`是FTP上传服务的实现类,其中`uploadFiles`方法使用了Java并发编程中的`ExecutorService`和`Future`来实现多个文件并行上传。`uploadFile`方法用于上传单个文件。
使用时,只需在Spring Boot的配置文件中添加FTP的相关配置,然后在需要上传文件的地方调用`FtpService`的`uploadFiles`方法即可。
需要注意的是,由于FTP的上传速度可能受到网络带宽等因素的影响,因此在上传大量文件时,建议适当控制并发数,以避免FTP服务器负载过高。
阅读全文
相关推荐
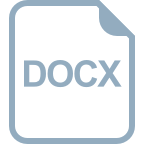
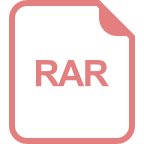

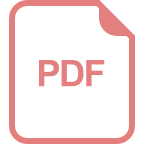
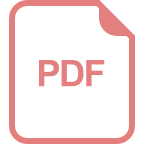
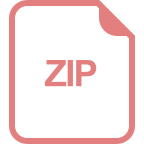
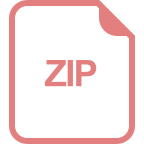
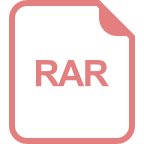
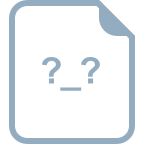
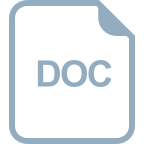
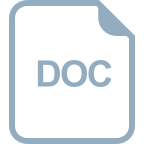
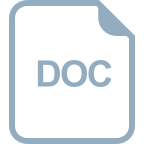
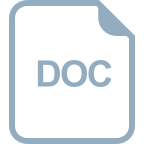
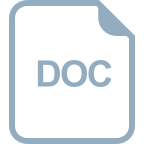
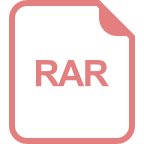
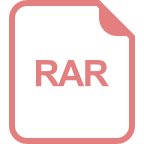