springboot上传文件到ftp
时间: 2023-04-27 09:05:08 浏览: 136
Spring Boot可以使用Apache Commons Net库来上传文件到FTP服务器。以下是一个简单的示例:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. 编写上传代码
在Spring Boot应用程序中,您可以使用以下代码将文件上传到FTP服务器:
```
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.io.InputStream;
@Service
public class FtpService {
private static final String FTP_HOST = "ftp.example.com";
private static final String FTP_USERNAME = "username";
private static final String FTP_PASSWORD = "password";
public void uploadFile(MultipartFile file) throws IOException {
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(FTP_HOST);
ftpClient.login(FTP_USERNAME, FTP_PASSWORD);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
String fileName = file.getOriginalFilename();
InputStream inputStream = file.getInputStream();
ftpClient.storeFile(fileName, inputStream);
} finally {
ftpClient.logout();
ftpClient.disconnect();
}
}
}
```
在上面的代码中,我们使用FTPClient类连接到FTP服务器,然后使用storeFile()方法将文件上传到服务器。
3. 测试上传
您可以使用以下代码测试上传:
```
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class FileUploadController {
@Autowired
private FtpService ftpService;
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
ftpService.uploadFile(file);
return "File uploaded successfully";
}
}
```
在上面的代码中,我们使用Spring MVC的@RequestParam注释将上传的文件作为参数传递给uploadFile()方法。
希望这可以帮助您将文件上传到FTP服务器。
阅读全文
相关推荐
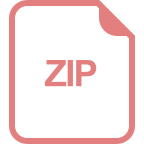


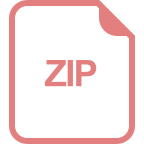
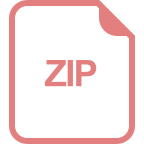
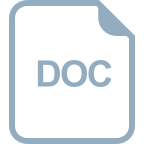
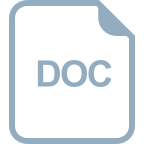
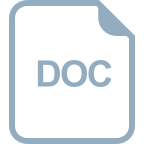
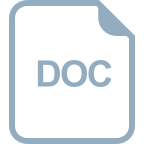
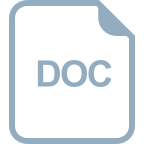
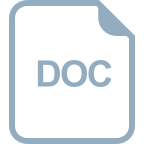





