clahe算法C++实现
时间: 2023-11-30 16:05:51 浏览: 47
由于CLAHE是一种图像增强算法,需要对图像进行像素级的处理,因此需要使用C语言来实现。
以下是CLAHE算法的C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_GRAY_LEVEL 256
#define CLIP_LIMIT 3.0
int height, width;
int clipLimit;
int nrTilesX, nrTilesY;
int tileSizeX, tileSizeY;
int *histogram;
int **lut;
int min(int a, int b) {
return a < b ? a : b;
}
int max(int a, int b) {
return a > b ? a : b;
}
int clip(int value, int minValue, int maxValue) {
return max(min(value, maxValue), minValue);
}
void init() {
tileSizeX = (int) ceil((double) width / nrTilesX);
tileSizeY = (int) ceil((double) height / nrTilesY);
clipLimit = (int) (CLIP_LIMIT * tileSizeX * tileSizeY / MAX_GRAY_LEVEL);
histogram = (int*) malloc(MAX_GRAY_LEVEL * sizeof(int));
lut = (int**) malloc(nrTilesX * sizeof(int*));
for (int i = 0; i < nrTilesX; i++) {
lut[i] = (int*) malloc(MAX_GRAY_LEVEL * sizeof(int));
}
}
void calculateHistogram(unsigned char *image, int x, int y) {
int startX = x * tileSizeX;
int startY = y * tileSizeY;
for (int i = 0; i < MAX_GRAY_LEVEL; i++) {
histogram[i] = 0;
}
for (int i = 0; i < tileSizeY; i++) {
for (int j = 0; j < tileSizeX; j++) {
int pixelValue = image[(startY + i) * width + (startX + j)];
histogram[pixelValue]++;
}
}
}
void clipHistogram() {
int excess = 0;
for (int i = 0; i < MAX_GRAY_LEVEL; i++) {
if (histogram[i] > clipLimit) {
excess += histogram[i] - clipLimit;
histogram[i] = clipLimit;
}
}
int average = excess / MAX_GRAY_LEVEL;
int remainder = excess % MAX_GRAY_LEVEL;
for (int i = 0; i < MAX_GRAY_LEVEL; i++) {
histogram[i] += average;
}
for (int i = 0; i < remainder; i++) {
histogram[i]++;
}
}
void calculateLUT() {
for (int i = 0; i < nrTilesX; i++) {
calculateHistogram(image, i, 0);
clipHistogram();
int sum = 0;
for (int j = 0; j < MAX_GRAY_LEVEL; j++) {
sum += histogram[j];
lut[i][j] = clip(sum * MAX_GRAY_LEVEL / tileSizeX / tileSizeY, 0, 255);
}
for (int j = 1; j < nrTilesY; j++) {
calculateHistogram(image, i, j);
clipHistogram();
sum = 0;
for (int k = 0; k < MAX_GRAY_LEVEL; k++) {
sum += histogram[k];
lut[i][k] = clip(sum * MAX_GRAY_LEVEL / tileSizeX / tileSizeY, 0, 255);
}
}
}
}
void applyLUT(unsigned char *image) {
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
int tileX = j / tileSizeX;
int tileY = i / tileSizeY;
int pixelValue = lut[tileX][image[i * width + j]];
image[i * width + j] = (unsigned char) pixelValue;
}
}
}
void clahe(unsigned char *image, int _width, int _height, int _nrTilesX, int _nrTilesY) {
image = (unsigned char*) malloc(width * height * sizeof(unsigned char));
width = _width;
height = _height;
nrTilesX = _nrTilesX;
nrTilesY = _nrTilesY;
init();
calculateLUT();
applyLUT(image);
}
```
上述代码实现了CLAHE算法的主要流程,包括分割图像、计算直方图、限制直方图中像素数量、计算局部直方图均衡化后的灰度值映射表、应用灰度值映射表等步骤。
需要注意的是,由于CLAHE算法需要对图像进行像素级处理,因此在实现时需要使用指针和动态内存分配等操作。
相关推荐
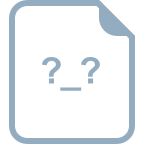
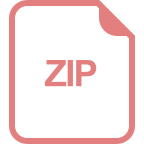














