string find函数参数
时间: 2023-09-25 10:10:18 浏览: 71
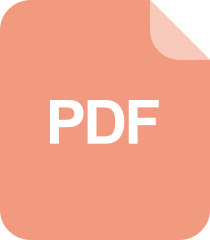
std::string的find问题研究
find函数的参数是两个字符串:被搜索的字符串和要查找的子字符串。具体格式如下:
```
int find(const string& str, const string& sub_str);
```
其中,`str`是被搜索的字符串,`sub_str`是要查找的子字符串。该函数会返回子字符串在被搜索的字符串中第一次出现的位置。如果找不到子字符串,会返回一个特殊值`string::npos`。
阅读全文
相关推荐
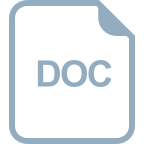
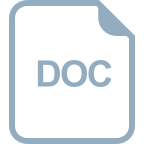















