#include <fstream> #include <iostream> using namespace std; void check( std::fstream &file ) { if( file.is_open() ) { cout<<"open"<<endl; } else { cout<<"close"<<endl; } } int main() { char buf[50] = {0}; std::fstream file("../caoxs.txt"); check( file ); file << "caoxs"; file >> buf; cout<<buf<<endl; file.close(); check( file ); return 0; } 为什么输出结果是 open close
时间: 2024-04-08 16:28:44 浏览: 44
在你的代码中,打开文件时使用的是 `std::fstream`,这是一个同时支持读写操作的文件流。当你调用 `check()` 函数时,如果文件成功打开,它会输出 "open",否则输出 "close"。
在 `file << "caoxs";` 这一行中,你使用输出运算符 `<<` 将字符串 "caoxs" 写入文件。这是一个写入操作。
然而,在 `file >> buf;` 这一行中,你试图使用输入运算符 `>>` 从文件中读取数据到缓冲区 `buf`。然而,请注意,`std::fstream` 并不支持直接使用输入运算符来读取数据,因此这一行代码并不能实现从文件中读取数据的功能。
由于没有成功从文件中读取数据,缓冲区 `buf` 的内容仍然是初始化时的空值。因此,输出结果为 "close",表示文件未成功打开。
如果你想从文件中读取数据到缓冲区 `buf`,你需要使用 `std::ifstream` 类型的对象来打开文件并进行读取操作,而不是 `std::fstream`。
以下是修改后的示例代码:
```cpp
#include <fstream>
#include <iostream>
using namespace std;
void check( std::ifstream &file )
{
if( file.is_open() )
{
cout << "open" << endl;
}
else
{
cout << "close" << endl;
}
}
int main()
{
char buf[50] = {0};
std::ifstream file("../caoxs.txt");
check( file );
file >> buf;
cout << buf << endl;
file.close();
check( file );
return 0;
}
```
在修改后的代码中,我们将 `std::fstream` 改为 `std::ifstream` 类型的对象,以便进行读取操作。这样,当文件成功打开时,`check()` 函数会输出 "open",然后我们使用输入运算符 `>>` 从文件中读取数据到缓冲区 `buf`。最后,我们关闭文件,并再次调用 `check()` 函数,输出结果应为 "close"。
阅读全文
相关推荐
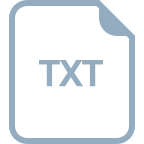
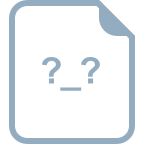
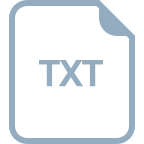
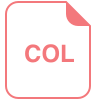
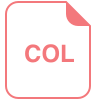
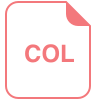
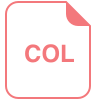
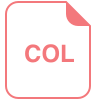


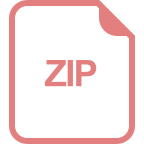