用c++写生命游戏,定义类cell,每个cell负责计算下一代的步数。并且细胞显示其状态
时间: 2024-03-18 12:39:19 浏览: 25
好的,下面是一个简单的 C++ 生命游戏实现,包含一个 `Cell` 类和一个 `LifeGame` 类,其中 `Cell` 负责计算下一代的状态,`LifeGame` 负责初始化游戏,并输出游戏状态。
Cell 类定义如下:
```c++
class Cell {
public:
Cell(int x, int y, bool alive = false);
void setAlive(bool alive);
bool isAlive() const;
int getX() const;
int getY() const;
int countNeighbors(const vector<Cell>& cells) const;
void nextGeneration(vector<Cell>& cells);
private:
int x_;
int y_;
bool alive_;
};
```
其中,`x_` 和 `y_` 表示细胞在生命游戏的坐标,`alive_` 表示细胞是否存活。`setAlive` 和 `isAlive` 分别用于设置和查询细胞的状态。`countNeighbors` 用于计算周围细胞的数量,`nextGeneration` 用于计算下一代细胞的状态。
LifeGame 类定义如下:
```c++
class LifeGame {
public:
LifeGame(int width, int height, int density);
void run();
private:
int width_;
int height_;
vector<Cell> cells_;
void updateCells();
void drawCells() const;
};
```
其中,`width_` 和 `height_` 表示生命游戏的大小,`density` 表示初始细胞的密度。`cells_` 存储生命游戏的所有细胞。`run` 用于运行生命游戏,`updateCells` 用于更新所有细胞的状态,`drawCells` 用于输出游戏状态。
下面是完整的实现代码:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
class Cell {
public:
Cell(int x, int y, bool alive = false);
void setAlive(bool alive);
bool isAlive() const;
int getX() const;
int getY() const;
int countNeighbors(const vector<Cell>& cells) const;
void nextGeneration(vector<Cell>& cells);
private:
int x_;
int y_;
bool alive_;
};
class LifeGame {
public:
LifeGame(int width, int height, int density);
void run();
private:
int width_;
int height_;
vector<Cell> cells_;
void updateCells();
void drawCells() const;
};
Cell::Cell(int x, int y, bool alive) : x_(x), y_(y), alive_(alive) {}
void Cell::setAlive(bool alive) {
alive_ = alive;
}
bool Cell::isAlive() const {
return alive_;
}
int Cell::getX() const {
return x_;
}
int Cell::getY() const {
return y_;
}
int Cell::countNeighbors(const vector<Cell>& cells) const {
int count = 0;
for (const auto& cell : cells) {
if (cell.isAlive() && (cell.getX() != x_ || cell.getY() != y_)) {
if (abs(cell.getX() - x_) <= 1 && abs(cell.getY() - y_) <= 1) {
count++;
}
}
}
return count;
}
void Cell::nextGeneration(vector<Cell>& cells) {
int neighbors = countNeighbors(cells);
if (alive_) {
if (neighbors < 2 || neighbors > 3) {
alive_ = false;
}
} else {
if (neighbors == 3) {
alive_ = true;
}
}
}
LifeGame::LifeGame(int width, int height, int density) : width_(width), height_(height) {
srand(time(nullptr));
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
if (rand() % 100 < density) {
cells_.emplace_back(i, j, true);
} else {
cells_.emplace_back(i, j, false);
}
}
}
}
void LifeGame::run() {
while (true) {
drawCells();
updateCells();
cout << "Press ENTER to continue, or q to quit." << endl;
char c = getchar();
if (c == 'q') {
break;
}
}
}
void LifeGame::updateCells() {
vector<Cell> nextCells;
for (auto& cell : cells_) {
cell.nextGeneration(cells_);
nextCells.push_back(cell);
}
cells_ = nextCells;
}
void LifeGame::drawCells() const {
system("clear");
for (int i = 0; i < width_; i++) {
for (int j = 0; j < height_; j++) {
bool found = false;
for (const auto& cell : cells_) {
if (cell.getX() == i && cell.getY() == j && cell.isAlive()) {
found = true;
break;
}
}
if (found) {
cout << "O";
} else {
cout << ".";
}
}
cout << endl;
}
}
int main() {
LifeGame game(50, 20, 30);
game.run();
return 0;
}
```
在这个实现中,`LifeGame` 构造函数接受三个参数:`width` 和 `height` 分别表示生命游戏的宽度和高度,`density` 表示初始细胞的密度。在构造函数中,我们使用 `rand()` 函数生成随机数,根据密度随机生成细胞。`run` 函数用于循环运行生命游戏,每次循环调用 `drawCells` 输出当前游戏状态,然后调用 `updateCells` 计算下一代细胞的状态。`drawCells` 函数用于输出游戏状态,使用 `.` 表示空白,使用 `O` 表示存活的细胞。`updateCells` 函数用于计算下一代细胞的状态,我们首先创建一个新的 `vector` 用于存储下一代细胞,然后遍历所有细胞,计算它们的下一代状态,并将结果存储在新的 `vector` 中,最后将新的 `vector` 赋值给 `cells_`。
这就是一个简单的 C++ 生命游戏实现,希望能对你有所帮助!
相关推荐
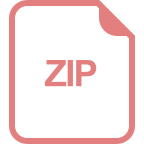
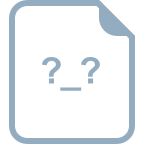
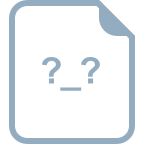








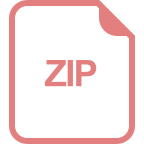
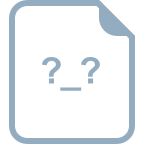
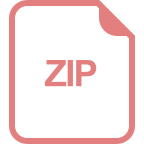
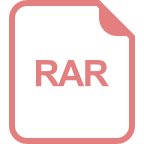
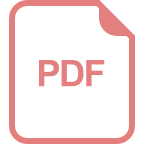
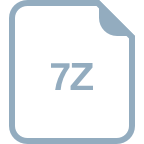