用c++写生命游戏,定义类cell,每个cell负责计算下一代的步数。并且细胞显示其状态
时间: 2024-03-18 21:39:02 浏览: 53
好的,我可以为你提供一份C++代码示例,用于实现生命游戏。
首先,我们需要定义一个 `Cell` 类,用于表示每个细胞。该类包含以下成员变量和成员函数:
```c++
class Cell {
private:
bool m_state; // 细胞状态,true表示细胞存活,false表示细胞死亡
int m_nextState; // 下一代状态,0表示死亡,1表示存活
int m_row; // 细胞所在的行数
int m_col; // 细胞所在的列数
int m_liveNeighbors; // 存活邻居数
public:
Cell(int row, int col); // 构造函数
void setState(bool state); // 设置细胞状态
bool getState() const; // 获取细胞状态
void setNextState(int state); // 设置下一代状态
int getNextState() const; // 获取下一代状态
void setRow(int row); // 设置细胞所在的行数
int getRow() const; // 获取细胞所在的行数
void setCol(int col); // 设置细胞所在的列数
int getCol() const; // 获取细胞所在的列数
void setLiveNeighbors(int count); // 设置存活邻居数
int getLiveNeighbors() const; // 获取存活邻居数
void calculateNextState(); // 计算下一代状态
void display() const; // 显示细胞状态
};
```
接下来,我们需要实现 `Cell` 类的成员函数。首先是构造函数:
```c++
Cell::Cell(int row, int col) {
m_state = false; // 默认细胞状态为死亡
m_nextState = 0; // 默认下一代状态为死亡
m_row = row;
m_col = col;
m_liveNeighbors = 0;
}
```
然后是设置和获取细胞状态的函数:
```c++
void Cell::setState(bool state) {
m_state = state;
}
bool Cell::getState() const {
return m_state;
}
```
设置和获取下一代状态的函数:
```c++
void Cell::setNextState(int state) {
m_nextState = state;
}
int Cell::getNextState() const {
return m_nextState;
}
```
设置和获取细胞所在行列数的函数:
```c++
void Cell::setRow(int row) {
m_row = row;
}
int Cell::getRow() const {
return m_row;
}
void Cell::setCol(int col) {
m_col = col;
}
int Cell::getCol() const {
return m_col;
}
```
设置和获取存活邻居数的函数:
```c++
void Cell::setLiveNeighbors(int count) {
m_liveNeighbors = count;
}
int Cell::getLiveNeighbors() const {
return m_liveNeighbors;
}
```
计算下一代状态的函数:
```c++
void Cell::calculateNextState() {
if (m_state) {
// 细胞存活
if (m_liveNeighbors <= 1 || m_liveNeighbors >= 4) {
// 存活邻居数小于等于1或大于等于4,细胞死亡
m_nextState = 0;
} else {
// 存活邻居数为2或3,细胞继续存活
m_nextState = 1;
}
} else {
// 细胞死亡
if (m_liveNeighbors == 3) {
// 存活邻居数为3,细胞复活
m_nextState = 1;
} else {
// 否则细胞继续死亡
m_nextState = 0;
}
}
}
```
最后是显示细胞状态的函数:
```c++
void Cell::display() const {
if (m_state) {
// 细胞存活,打印'*'
std::cout << "*";
} else {
// 细胞死亡,打印'.'
std::cout << ".";
}
}
```
现在我们已经实现了 `Cell` 类,接下来是实现整个生命游戏的逻辑。我们可以使用一个二维数组来表示整个生命游戏的状态,然后在每一轮迭代中,遍历所有细胞,计算每个细胞的下一代状态,并将其保存到二维数组中。代码如下:
```c++
const int ROWS = 10; // 行数
const int COLS = 10; // 列数
int main() {
// 初始化细胞状态
Cell cells[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = Cell(i, j);
cells[i][j].setState(rand() % 2 == 0); // 随机生成细胞状态
}
}
// 迭代10轮
for (int k = 0; k < 10; k++) {
// 计算每个细胞的存活邻居数
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int liveNeighbors = 0;
for (int m = i - 1; m <= i + 1; m++) {
for (int n = j - 1; n <= j + 1; n++) {
if (m == i && n == j) continue; // 排除自身
if (m < 0 || m >= ROWS || n < 0 || n >= COLS) continue; // 排除越界的细胞
if (cells[m][n].getState()) liveNeighbors++; // 统计存活邻居数
}
}
cells[i][j].setLiveNeighbors(liveNeighbors); // 保存存活邻居数
}
}
// 计算每个细胞的下一代状态
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j].calculateNextState(); // 计算下一代状态
}
}
// 更新细胞状态
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j].setState(cells[i][j].getNextState()); // 更新细胞状态
}
}
// 显示当前生命游戏状态
std::cout << "Generation " << k << ":" << std::endl;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j].display();
}
std::cout << std::endl;
}
std::cout << std::endl;
}
return 0;
}
```
这段代码会迭代10轮生命游戏,并在每一轮结束后打印当前生命游戏状态。你可以根据需要自行修改行数、列数以及迭代轮数。
希望这份代码示例能够对你有所帮助!
阅读全文
相关推荐
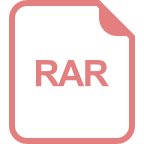
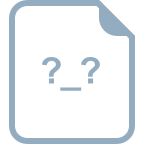




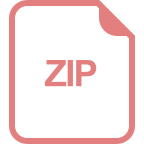
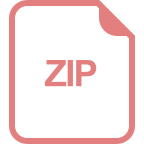
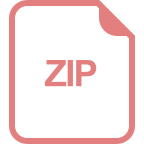
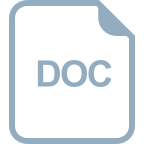





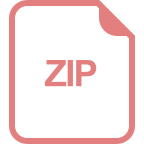