如果一个字符串正着读和反着读都一样,那它就是回文串。请写一个函数,参数为个字符串,返回这个字符串所有子串里面构成回文串的最大子串v
时间: 2024-06-17 11:06:32 浏览: 13
好的,可以使用中心扩展算法来解决这个问题。具体做法是从字符串的第一个字符开始,以该字符为中心向两边扩展,记录下能够扩展的最长回文子串的起始和结束位置,同时向后移动中心位置,重复以上操作,最后得到所有的回文子串中最长的一个。
以下是具体实现代码:
```python
def longest_palindrome(s: str) -> str:
start, end = 0, 0
for i in range(len(s)):
# 以当前字符为中心向两边扩展
len1 = expand_around_center(s, i, i)
# 以当前字符和下一个字符之间为中心向两边扩展
len2 = expand_around_center(s, i, i + 1)
# 取两种情况下最长的回文子串
max_len = max(len1, len2)
# 如果当前回文子串更长,则更新起始和结束位置
if max_len > end - start:
start = i - (max_len - 1) // 2
end = i + max_len // 2
return s[start:end+1]
def expand_around_center(s: str, left: int, right: int) -> int:
# 中心扩展
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
```
其中,expand_around_center函数用于以指定位置为中心向两边扩展,返回最长回文子串的长度;longest_palindrome函数则用于找出所有回文子串中最长的一个。时间复杂度为O(n^2)。
相关推荐
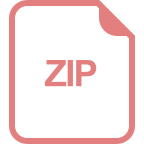
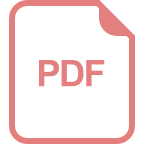
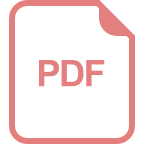














