用C++实现双端队列
时间: 2024-06-13 09:09:07 浏览: 170
以下是用C++实现双端队列的代码和详细步骤:
```cpp
#include <iostream>
using namespace std;
#define MAXSIZE 100 // 定义双端队列的最大长度
class Deque {
private:
int data[MAXSIZE]; // 双端队列的数据存储数组
int left; // 左指针
int right; // 右指针
public:
Deque() { // 构造函数,初始化左右指针
left = 0;
right = 0;
}
bool isEmpty() { // 判断队列是否为空
return left == right;
}
bool isFull() { // 判断队列是否已满
return (right + 1) % MAXSIZE == left;
}
void push_front(int x) { // 在队头插入元素
if (isFull()) {
cout << "Deque is full!" << endl;
return;
}
left = (left - 1 + MAXSIZE) % MAXSIZE;
data[left] = x;
}
void push_back(int x) { // 在队尾插入元素
if (isFull()) {
cout << "Deque is full!" << endl;
return;
}
data[right] = x;
right = (right + 1) % MAXSIZE;
}
void pop_front() { // 在队头删除元素
if (isEmpty()) {
cout << "Deque is empty!" << endl;
return;
}
left = (left + 1) % MAXSIZE;
}
void pop_back() { // 在队尾删除元素
if (isEmpty()) {
cout << "Deque is empty!" << endl;
return;
}
right = (right - 1 + MAXSIZE) % MAXSIZE;
}
int front() { // 返回队头元素
if (isEmpty()) {
cout << "Deque is empty!" << endl;
return -1;
}
return data[left];
}
int back() { // 返回队尾元素
if (isEmpty()) {
cout << "Deque is empty!" << endl;
return -1;
}
return data[(right - 1 + MAXSIZE) % MAXSIZE];
}
};
int main() {
Deque dq;
dq.push_front(1);
dq.push_back(2);
dq.push_front(3);
dq.push_back(4);
cout << dq.front() << endl; // 输出:3
cout << dq.back() << endl; // 输出:4
dq.pop_front();
dq.pop_back();
cout << dq.front() << endl; // 输出:1
cout << dq.back() << endl; // 输出:2
return 0;
}
```
步骤:
1. 定义一个常量MAXSIZE,表示双端队列的最大长度。
2. 定义一个类Deque,包含数据存储数组data和左右指针left、right。
3. 构造函数初始化左右指针。
4. 定义isEmpty()和isFull()函数,分别判断队列是否为空和已满。
5. 定义push_front()和push_back()函数,在队头和队尾插入元素。
6. 定义pop_front()和pop_back()函数,在队头和队尾删除元素。
7. 定义front()和back()函数,分别返回队头和队尾元素。
8. 在main函数中创建Deque对象dq,测试双端队列的各种操作。
阅读全文
相关推荐
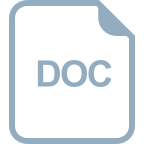
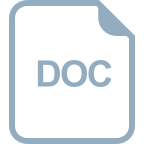
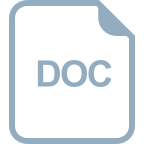



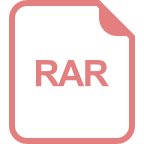
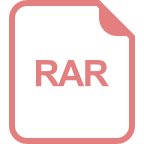
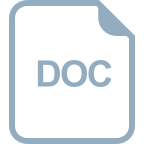
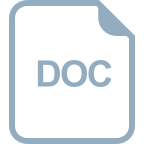
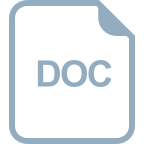
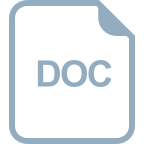
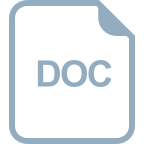
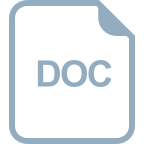


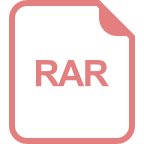