写c语言图书管理系统程序
时间: 2023-11-30 13:59:34 浏览: 30
以下是一个简单的 C 语言图书管理系统程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[50];
char author[50];
int year;
};
struct Library {
struct Book books[MAX_BOOKS];
int num_books;
};
void display_menu() {
printf("Welcome to the Library Management System\n");
printf("----------------------------------------\n");
printf("1. Add book\n");
printf("2. Remove book\n");
printf("3. Display all books\n");
printf("4. Search book by title\n");
printf("5. Search book by author\n");
printf("6. Exit\n");
}
void add_book(struct Library *library) {
if (library->num_books >= MAX_BOOKS) {
printf("Error: library is full\n");
return;
}
printf("Enter book title: ");
scanf("%s", library->books[library->num_books].title);
printf("Enter book author: ");
scanf("%s", library->books[library->num_books].author);
printf("Enter book year: ");
scanf("%d", &library->books[library->num_books].year);
library->num_books++;
printf("Book added successfully\n");
}
void remove_book(struct Library *library) {
char title[50];
printf("Enter book title to remove: ");
scanf("%s", title);
for (int i = 0; i < library->num_books; i++) {
if (strcmp(title, library->books[i].title) == 0) {
for (int j = i; j < library->num_books - 1; j++) {
strcpy(library->books[j].title, library->books[j+1].title);
strcpy(library->books[j].author, library->books[j+1].author);
library->books[j].year = library->books[j+1].year;
}
library->num_books--;
printf("Book removed successfully\n");
return;
}
}
printf("Error: book not found\n");
}
void display_books(struct Library *library) {
printf("%-30s %-30s %-4s\n", "Title", "Author", "Year");
printf("----------------------------------------\n");
for (int i = 0; i < library->num_books; i++) {
printf("%-30s %-30s %-4d\n", library->books[i].title, library->books[i].author, library->books[i].year);
}
}
void search_book_by_title(struct Library *library) {
char title[50];
printf("Enter book title to search: ");
scanf("%s", title);
for (int i = 0; i < library->num_books; i++) {
if (strcmp(title, library->books[i].title) == 0) {
printf("%-30s %-30s %-4s\n", "Title", "Author", "Year");
printf("----------------------------------------\n");
printf("%-30s %-30s %-4d\n", library->books[i].title, library->books[i].author, library->books[i].year);
return;
}
}
printf("Error: book not found\n");
}
void search_book_by_author(struct Library *library) {
char author[50];
printf("Enter book author to search: ");
scanf("%s", author);
for (int i = 0; i < library->num_books; i++) {
if (strcmp(author, library->books[i].author) == 0) {
printf("%-30s %-30s %-4s\n", "Title", "Author", "Year");
printf("----------------------------------------\n");
printf("%-30s %-30s %-4d\n", library->books[i].title, library->books[i].author, library->books[i].year);
}
}
printf("Error: book not found\n");
}
int main() {
struct Library library = {0};
int choice;
do {
display_menu();
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book(&library);
break;
case 2:
remove_book(&library);
break;
case 3:
display_books(&library);
break;
case 4:
search_book_by_title(&library);
break;
case 5:
search_book_by_author(&library);
break;
case 6:
printf("Goodbye!\n");
break;
default:
printf("Error: invalid choice\n");
break;
}
} while (choice != 6);
return 0;
}
```
注意:这只是一个简单的示例程序,实际的图书管理系统需要更全面和复杂的功能。
相关推荐
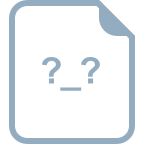














