怎么用c语言代码将哈夫曼编码转换成对应字符串
时间: 2023-11-12 20:05:01 浏览: 102
要将哈夫曼编码转换成对应字符串,你需要进行以下步骤:
1. 定义哈夫曼编码表:哈夫曼编码表是一个数组,其中每个元素对应一个字符及其对应的哈夫曼编码。
2. 读取哈夫曼编码:从文件或其他数据源中读取哈夫曼编码。
3. 解码哈夫曼编码:将读取到的哈夫曼编码按位进行解码,根据哈夫曼编码表中的对应关系,将每个编码对应的字符拼接成字符串。
下面是一个示例的 C 语言代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_CODE_LEN 50
// 哈夫曼编码表
struct HuffmanCode {
char ch;
char code[MAX_CODE_LEN];
};
// 将哈夫曼编码转换成字符串
void decode_huffman_code(char *code, struct HuffmanCode *huffman_codes, char *str) {
int i = 0, j = 0;
while (code[i] != '\0') {
char tmp[MAX_CODE_LEN] = "";
while (code[i] != '0' && code[i] != '1') {
i++;
}
while (code[i] == '0' || code[i] == '1') {
str[j++] = code[i++];
str[j] = '\0'; // 在末尾添加结束符
}
for (int k = 0; k < strlen(str); k++) {
strcat(tmp, &str[k]); // 将字符串逐个字符拼接
for (int l = 0; l < 26; l++) { // 在哈夫曼编码表中查找对应字符
if (strcmp(tmp, huffman_codes[l].code) == 0) {
str[j - strlen(str)] = huffman_codes[l].ch;
break;
}
}
}
}
}
int main() {
struct HuffmanCode huffman_codes[26] = { // 假设只有 26 个小写字母需要编码
{'a', "010"},
{'b', "011"},
{'c', "100"},
{'d', "101"},
{'e', "110"},
{'f', "1110"},
{'g', "11110"},
{'h', "111110"},
{'i', "1111110"},
{'j', "11111110"},
{'k', "111111110"},
{'l', "1111111110"},
{'m', "11111111110"},
{'n', "111111111110"},
{'o', "1111111111110"},
{'p', "11111111111110"},
{'q', "111111111111110"},
{'r', "1111111111111110"},
{'s', "11111111111111110"},
{'t', "111111111111111110"},
{'u', "1111111111111111110"},
{'v', "11111111111111111110"},
{'w', "111111111111111111110"},
{'x', "1111111111111111111110"},
{'y', "11111111111111111111110"},
{'z', "111111111111111111111110"}
};
char code[] = "011110101101110111011111011111110111111111010101110111111111111000101111111111111111111111111110";
char str[MAX_CODE_LEN] = "";
decode_huffman_code(code, huffman_codes, str);
printf("Decoded string: %s\n", str);
return 0;
}
```
在这个示例代码中,我们定义了一个包含 26 个小写字母的哈夫曼编码表,并且读取了一个示例编码。在 `decode_huffman_code()` 函数中,我们对哈夫曼编码进行解码,并在哈夫曼编码表中查找对应的字符,将其拼接成字符串。最后,我们将解码后的字符串输出。
阅读全文
相关推荐
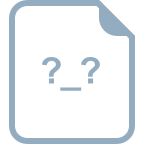

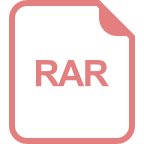
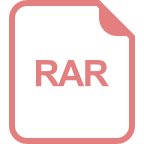
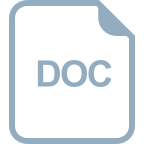

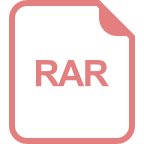
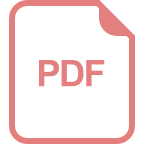
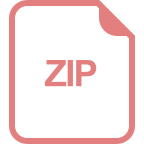
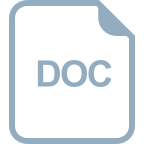
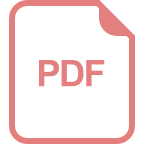
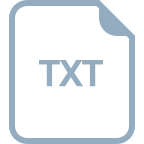
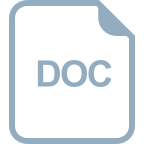
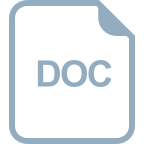


