Python拼图小游戏
时间: 2023-07-02 14:03:40 浏览: 116
好的,下面是一个实现 Python 拼图小游戏的例子。游戏规则是将九个数字拼成一个 3x3 的方格,其中一个位置是空位,可以通过移动数字来完成拼图。
```python
import random
# 初始化游戏状态
board = [[1, 2, 3], [4, 5, 6], [7, 8, None]]
# 交换指定位置和空位的数字
def move(row, col):
if 0 <= row < 3 and 0 <= col < 3 and board[row][col] is not None:
for i, row2 in enumerate(board):
for j, val in enumerate(row2):
if val is None:
board[row][col], board[i][j] = board[i][j], board[row][col]
return True
return False
# 判断是否完成拼图
def is_solved():
return all(board[i][j] == i * 3 + j + 1 for i in range(3) for j in range(2)) and board[2][2] is None
# 打印游戏状态
def print_board():
for row in board:
for val in row:
print(f"{val:2d}" if val is not None else " ", end=" ")
print()
# 随机打乱游戏状态
for i in range(100):
move(random.randint(0, 2), random.randint(0, 2))
# 游戏循环
while True:
print_board()
if is_solved():
print("You win!")
break
row, col = map(int, input("Enter row and column: ").split())
if not move(row, col):
print("Invalid move.")
```
这个例子使用一个二维列表 `board` 来保存游戏状态,其中 `None` 表示空位。`move` 函数接受一个行列坐标,如果该坐标处有数字且可以和空位交换,则交换它们并返回 `True`。`is_solved` 函数检查是否完成拼图,即每个数字都在正确的位置上,且空位在右下角。`print_board` 函数将游戏状态打印出来,其中使用了格式化字符串来保证每个数字占两个字符宽度。最后,使用 `random.randint` 函数随机打乱游戏状态,并使用一个简单的循环让用户输入行列坐标来移动数字,直到完成拼图。
阅读全文
相关推荐
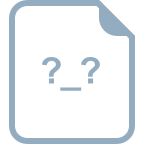
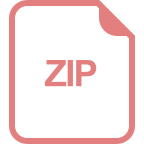



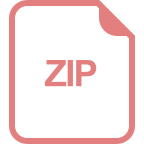
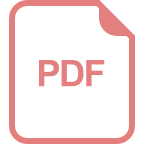
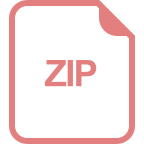
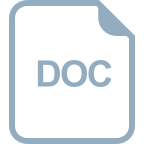
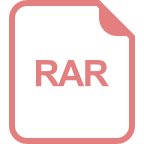





