帮我生成一段python代码,贪吃蛇
时间: 2023-10-22 22:12:36 浏览: 38
好的,以下是一个简单的Python贪吃蛇游戏代码:
```
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WIDTH = 800
HEIGHT = 600
win = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Snake Game")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 定义贪吃蛇类
class Snake():
def __init__(self):
self.body = [(250, 250), (240, 250), (230, 250), (220, 250)]
self.direction = "RIGHT"
def move(self):
if self.direction == "RIGHT":
new_head = (self.body[0][0] + 10, self.body[0][1])
elif self.direction == "LEFT":
new_head = (self.body[0][0] - 10, self.body[0][1])
elif self.direction == "UP":
new_head = (self.body[0][0], self.body[0][1] - 10)
elif self.direction == "DOWN":
new_head = (self.body[0][0], self.body[0][1] + 10)
self.body.insert(0, new_head)
self.body.pop()
def change_direction(self, direction):
if self.direction == "UP" and direction != "DOWN":
self.direction = direction
elif self.direction == "DOWN" and direction != "UP":
self.direction = direction
elif self.direction == "LEFT" and direction != "RIGHT":
self.direction = direction
elif self.direction == "RIGHT" and direction != "LEFT":
self.direction = direction
def draw(self, win):
for part in self.body:
pygame.draw.rect(win, GREEN, (part[0], part[1], 10, 10))
# 定义食物类
class Food():
def __init__(self):
self.x = random.randint(0, WIDTH - 10)
self.y = random.randint(0, HEIGHT - 10)
def draw(self, win):
pygame.draw.rect(win, RED, (self.x, self.y, 10, 10))
# 初始化游戏
def game():
snake = Snake()
food = Food()
clock = pygame.time.Clock()
# 游戏循环
while True:
# 处理用户输入
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.change_direction("UP")
elif event.key == pygame.K_DOWN:
snake.change_direction("DOWN")
elif event.key == pygame.K_LEFT:
snake.change_direction("LEFT")
elif event.key == pygame.K_RIGHT:
snake.change_direction("RIGHT")
# 移动贪吃蛇
snake.move()
# 检查是否吃到食物
if snake.body[0][0] == food.x and snake.body[0][1] == food.y:
food = Food()
snake.body.append(snake.body[-1])
# 检查游戏是否结束
if snake.body[0][0] < 0 or snake.body[0][0] > WIDTH - 10 or snake.body[0][1] < 0 or snake.body[0][1] > HEIGHT - 10:
print("Game Over!")
pygame.quit()
quit()
# 绘制游戏界面
win.fill(BLACK)
snake.draw(win)
food.draw(win)
pygame.display.update()
# 控制游戏帧数
clock.tick(15)
# 运行游戏
game()
```
希望这个代码可以帮助到你,有任何问题欢迎随时提出。
相关推荐
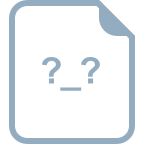
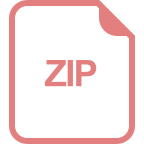










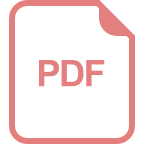
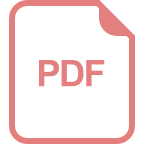
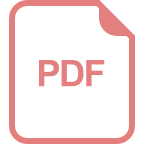
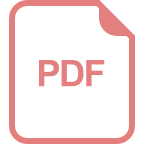