c++ 头文件使用unordered_map报错
时间: 2023-11-24 14:51:50 浏览: 98
根据提供的引用内容,如果使用C++11中的unordered_map头文件报错,可以尝试以下两种方法解决:
1. 修改编译配置,将C++标准设置为c++11,具体方法是将鼠标移动到出错的位置,选择修改configurations,然后将C++标准设置为c++11即可。
2. 手动添加头文件#include<unordered_map>,如果使用了bits/stdc++.h头文件,可能会导致无法检索到unordered_map,此时需要手动添加该头文件。
相关问题
c++ 为什么引入unordered_map头文件后说unordered_map不是模板
unordered_map 是 C++ STL 中的一个模板类,用于实现哈希表。在使用 unordered_map 时,需要引入 `<unordered_map>` 头文件,并使用命名空间 std。如果你在引入头文件后仍然提示 "unordered_map 不是模板",可能是因为编译器不支持或未正确配置 C++11 标准。请检查编译器版本和相关配置,确保支持 C++11 或更高版本的标准。
c++ 在头文件中使用unordered_map
在C++中,使用unordered_map需要包含头文件<unordered_map>。下面是一个使用unordered_map的例子:
```c++
#include <iostream>
#include <unordered_map>
using namespace std;
int main() {
unordered_map<string, int> umap;
umap["apple"] = 1;
umap["banana"] = 2;
umap["cherry"] = 3;
cout << "The value of apple is: " << umap["apple"] << endl;
cout << "The value of banana is: " << umap["banana"] << endl;
cout << "The value of cherry is: " << umap["cherry"] << endl;
return 0;
}
```
输出结果为:
```
The value of apple is: 1
The value of banana is: 2
The value of cherry is: 3
```
阅读全文
相关推荐
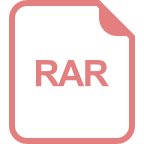













