c字符串队列
时间: 2023-08-18 16:05:04 浏览: 260
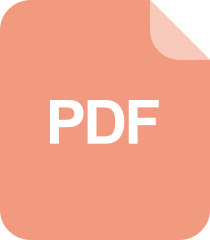
C 字符串
一个C字符串队列(String Queue)是一个基于FIFO(先进先出)原则的数据结构,其中存储了多个字符串。在C语言中,我们可以使用指针数组来实现该数据结构。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_QUEUE_SIZE 100
char* queue[MAX_QUEUE_SIZE];
int front = 0;
int rear = -1;
int itemCount = 0;
int isEmpty() {
return itemCount == 0;
}
int isFull() {
return itemCount == MAX_QUEUE_SIZE;
}
int size() {
return itemCount;
}
void insert(char* data) {
if(!isFull()) {
if(rear == MAX_QUEUE_SIZE-1) {
rear = -1;
}
queue[++rear] = malloc(strlen(data)+1); // Allocate memory for the string
strcpy(queue[rear], data); // Copy the string into the allocated memory
itemCount++;
}
}
char* removeData() {
if(!isEmpty()) {
char* data = queue[front++];
if(front == MAX_QUEUE_SIZE) {
front = 0;
}
itemCount--;
return data;
} else {
return NULL;
}
}
int main() {
insert("Hello");
insert("World");
insert("How");
insert("Are");
insert("You");
while(!isEmpty()) {
char* data = removeData();
printf("%s ", data);
free(data); // Free the memory allocated for the string
}
return 0;
}
```
在该示例代码中,我们使用了一个指针数组`queue`来存储字符串。`front`和`rear`分别表示队列的前端和后端位置。`itemCount`表示队列中元素的数量。`isEmpty()`和`isFull()`函数分别用于检查队列是否为空和是否已满。`insert()`函数用于向队列中插入字符串,`removeData()`函数用于从队列中移除字符串。`size()`函数返回队列中元素的数量。
在`insert()`函数中,我们首先检查队列是否已满。如果队列未满,则将字符串复制到新分配的内存中,并将指针存储到`queue`数组中。然后,`rear`变量增加1,表示队列的末尾位置已经发生了改变。`itemCount`变量也会增加1。
在`removeData()`函数中,我们首先检查队列是否为空。如果队列不为空,则将指向队列前端的指针存储到`data`变量中。然后,`front`变量增加1,表示队列前端位置已经改变。`itemCount`变量也会减少1。最后,我们返回`data`变量中存储的指针。
需要注意的是,在使用完字符串后,我们需要使用`free()`函数释放为该字符串分配的内存,以避免内存泄漏。
阅读全文
相关推荐
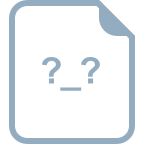

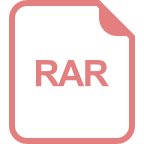
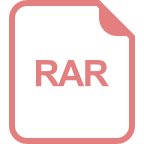




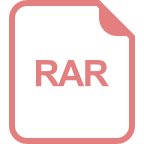







