在串中使用指针同时实现bf算法和kmp算法的代码
时间: 2024-12-08 13:15:35 浏览: 16
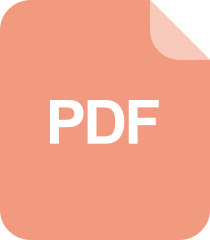
字符串的模式匹配详解--BF算法与KMP算法

在字符串匹配中,Boyer-Moore(BF)算法和Knuth-Morris-Pratt(KMP)算法都是常用的模式搜索技术。这两种算法虽然功能类似,但实现方式不同。Boyer-Moore算法主要依赖于坏字符规则和好后缀规则,而KMP算法则通过创建一个部分匹配表(也称为失步字典)来避免回溯。
如果你想同时展示如何在字符串中使用指针实现这两种算法,下面是一个简化版的KMP算法示例,因为它更适合利用指针:
```c++
#include <vector>
#include <string>
// KMP的部分匹配表生成函数
std::vector<int> getPartialMatchTable(const std::string& pattern) {
int table[pattern.size()];
table[0] = -1;
for (int i = 1, j = -1; i < pattern.size(); ++i) {
while (j != -1 && pattern[i] != pattern[j + 1]) {
j = table[j];
}
if (pattern[i] == pattern[j + 1]) {
++j;
}
table[i] = j;
}
return table;
}
// 使用指针的KMP主函数
bool kmpSearch(const std::string& text, const std::string& pattern) {
std::vector<int> table = getPartialMatchTable(pattern);
int i = 0, j = 0;
while (i < text.size()) {
if (text[i] == pattern[j]) {
++i;
++j;
} else if (j != 0) {
j = table[j - 1]; // 如果当前字符不符,跳转到失步位置
} else {
++i; // 如果整个模式都不符,文本移动一位
}
if (j == pattern.size()) {
return true; // 找到了匹配
}
}
return false; // 没有找到匹配
}
阅读全文
相关推荐
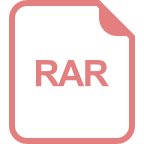
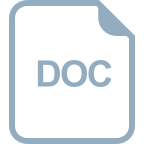
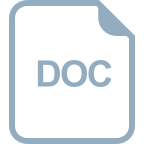







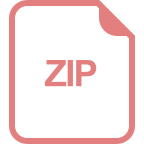
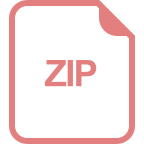





