java创建mapping_ElasticSearch使用Java客户端创建索引index和映射mapping
时间: 2023-12-24 22:04:41 浏览: 127
可以使用Java客户端创建索引index和映射mapping,下面是示例代码:
```java
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.action.admin.indices.create.CreateIndexResponse;
import org.elasticsearch.action.admin.indices.mapping.put.PutMappingRequest;
import org.elasticsearch.action.admin.indices.mapping.put.PutMappingResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentBuilder;
import org.elasticsearch.common.xcontent.XContentFactory;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.mapper.ObjectMapper;
import org.elasticsearch.index.mapper.ObjectMapper.Builder;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class CreateIndexAndMapping {
private RestHighLevelClient client;
public CreateIndexAndMapping(RestHighLevelClient client) {
this.client = client;
}
public void createIndex(String indexName) throws IOException {
CreateIndexRequest request = new CreateIndexRequest(indexName);
CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT);
boolean acknowledged = response.isAcknowledged();
if (acknowledged) {
System.out.println("Index created successfully.");
}
}
public void createMapping(String indexName, String typeName) throws IOException {
PutMappingRequest request = new PutMappingRequest(indexName);
request.type(typeName);
XContentBuilder builder = XContentFactory.jsonBuilder();
builder.startObject();
{
builder.startObject("properties");
{
builder.startObject("title");
{
builder.field("type", "text");
}
builder.endObject();
builder.startObject("author");
{
builder.field("type", "text");
}
builder.endObject();
builder.startObject("publish_date");
{
builder.field("type", "date");
}
builder.endObject();
builder.startObject("price");
{
builder.field("type", "double");
}
builder.endObject();
builder.startObject("description");
{
builder.field("type", "text");
}
builder.endObject();
}
builder.endObject();
}
builder.endObject();
request.source(builder);
PutMappingResponse response = client.indices().putMapping(request, RequestOptions.DEFAULT);
boolean acknowledged = response.isAcknowledged();
if (acknowledged) {
System.out.println("Mapping created successfully.");
}
}
}
```
上述代码中,`createIndex`方法用于创建索引,`createMapping`方法用于创建映射。在`createMapping`方法中,我们使用`XContentBuilder`来构建映射的JSON格式,然后将其作为请求的source进行设置。在这个例子中,我们定义了一个包含`title`、`author`、`publish_date`、`price`和`description`等字段的文档。
阅读全文
相关推荐
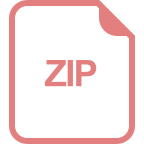
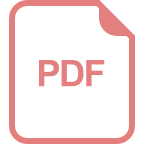
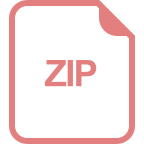
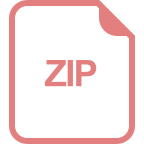





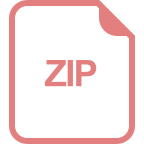
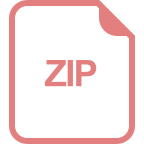
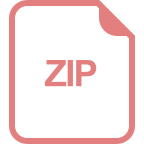




