stm32按键控制led灯延时1s灭
时间: 2023-09-16 14:14:51 浏览: 184
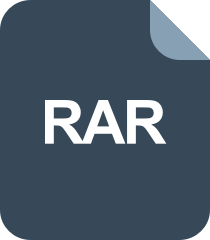
stm32f407zet6---自动灯光调节(光敏电阻),stm32f407按键控制led灯,C,C++

假设你使用的是STM32F4开发板,以下是一个简单的代码示例,可以通过按下按键控制LED灯的状态,并且在LED灯亮起后延时1秒再熄灭。
首先,需要定义LED灯引脚和按键引脚:
```c
#define LED_PIN GPIO_Pin_13
#define LED_GPIO_PORT GPIOD
#define LED_GPIO_CLK RCC_AHB1Periph_GPIOD
#define KEY_PIN GPIO_Pin_0
#define KEY_GPIO_PORT GPIOA
#define KEY_GPIO_CLK RCC_AHB1Periph_GPIOA
```
然后,需要初始化GPIO口:
```c
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable the GPIO_LED Clock */
RCC_AHB1PeriphClockCmd(LED_GPIO_CLK, ENABLE);
/* Configure the GPIO_LED pin */
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO_PORT, &GPIO_InitStructure);
/* Enable the GPIO_KEY Clock */
RCC_AHB1PeriphClockCmd(KEY_GPIO_CLK, ENABLE);
/* Configure the GPIO_KEY pin */
GPIO_InitStructure.GPIO_Pin = KEY_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_DOWN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(KEY_GPIO_PORT, &GPIO_InitStructure);
```
接下来,可以编写一个函数来实现延时:
```c
void delay(uint32_t time) {
uint32_t i;
for (i = 0; i < time; i++) {
__NOP();
}
}
```
最后,可以编写主循环来控制LED灯的状态和延时:
```c
while (1) {
if (GPIO_ReadInputDataBit(KEY_GPIO_PORT, KEY_PIN) == Bit_SET) {
/* Turn on LED */
GPIO_SetBits(LED_GPIO_PORT, LED_PIN);
/* Delay for 1 second */
delay(8400000);
/* Turn off LED */
GPIO_ResetBits(LED_GPIO_PORT, LED_PIN);
}
}
```
在这个代码中,当按键被按下时,LED灯会亮起,并且延时1秒后熄灭。你可以根据自己的需要修改延时时间。
阅读全文
相关推荐


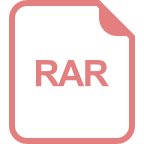
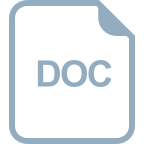
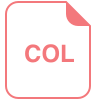










