keil5rs485接受数组
时间: 2023-07-29 10:07:02 浏览: 177
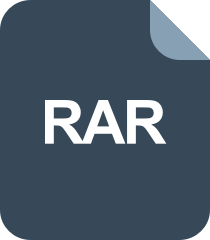
基于keil环境下, RS485通讯测试
要在Keil中使用RS485接收数组,需要以下步骤:
1. 设置串口通信参数,例如波特率、数据位、停止位等。
2. 在代码中定义一个存储接收数据的数组。
3. 在串口接收中断处理函数中,将接收到的数据存储到数组中。
以下是一个基本的示例代码:
```c
#include <stdio.h>
#include <stdint.h>
#include "stm32f10x.h"
#define MAX_RX_BUF_SIZE 256
uint8_t rx_buf[MAX_RX_BUF_SIZE];
volatile uint16_t rx_buf_index = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
rx_buf[rx_buf_index++] = USART_ReceiveData(USART1);
if (rx_buf_index >= MAX_RX_BUF_SIZE)
{
rx_buf_index = 0;
}
}
}
int main()
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// Set up USART
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_Cmd(USART1, ENABLE);
// Set up NVIC
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
while(1)
{
// Do something
}
}
```
在上面的代码中,我们定义了一个名为rx_buf的数组来存储接收到的数据。在USART1_IRQHandler中断处理函数中,将接收到的数据存储到rx_buf数组中,并且通过检查rx_buf_index是否超过最大缓冲区大小来避免数组溢出。在主函数中,我们可以在while循环中执行其他任务。
阅读全文
相关推荐
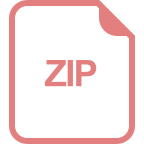
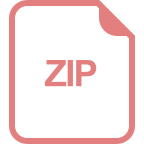




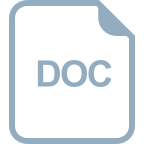
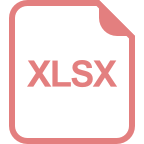
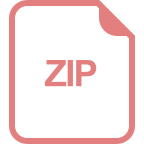
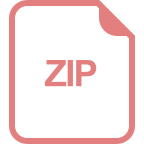
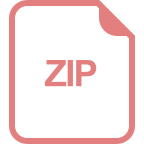
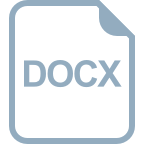
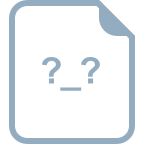
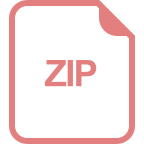
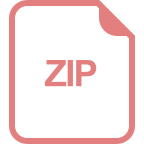
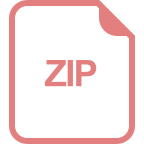