用c++实现字符串问题:设计一个通用函数char *insert(char *s1,char *s2,int n)实现在字符串s1中从第m个字符开始插入字符串s2,在主程序中对该函数进行测试。
时间: 2024-09-30 19:05:13 浏览: 21
在C++中,你可以通过动态内存分配和指针操作来实现这个功能。首先,你需要创建一个新的字符串,将`s1`的前`m-1`个字符复制到新字符串,然后添加`s2`的内容,最后将剩余的`s1`的字符追加到新字符串的末尾。以下是简单的步骤:
```cpp
#include <cstring>
using namespace std;
// 定义插入函数
char* insert(char* s1, char* s2, int m, int n) {
if (s1 == nullptr || s2 == nullptr) return nullptr;
int len1 = strlen(s1);
int len2 = strlen(s2);
// 计算新的总长度
int total_len = len1 + n + (len2 - 1); // 减一是因为要在s1的第m个位置插入
// 分配足够的空间
char* new_str = new char[total_len + 1]; // 空间用于新字符串,并额外加1位用于'\0'结束符
// 复制s1的前m-1个字符
if (m > 0) {
strncpy(new_str, s1, m - 1);
}
// 插入s2
memcpy(new_str + (m - 1), s2, n);
// 将剩余的s1追加到new_str的末尾
if (m + n <= len1) {
memcpy(new_str + m + n, s1 + m, len1 - m - n);
} else {
copy(s1 + m, s1 + len1, new_str + m + n);
}
// 结束符
new_str[total_len] = '\0';
return new_str;
}
int main() {
char* s1 = "Hello";
char* s2 = "World";
int m = 6; // 在's1'的第6个字符开始插入
int n = 5; // 插入的字符串长度
char* result = insert(s1, s2, m, n);
if (result != nullptr) {
cout << "Result: " << result << endl;
delete[] result; // 释放内存
} else {
cout << "Error: Insertion failed." << endl;
}
阅读全文
相关推荐
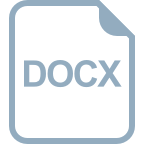
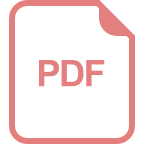
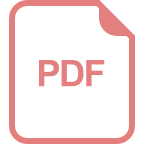













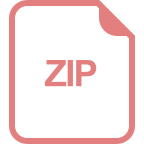