如何用c语言实现img2col算法卷积
时间: 2024-05-04 16:20:29 浏览: 13
Img2col是将输入图像转换为矩阵形式,便于矩阵乘法计算卷积。下面是用C语言实现img2col算法卷积的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义卷积核大小
#define KERNEL_SIZE 3
// 定义输入图像大小
#define INPUT_HEIGHT 5
#define INPUT_WIDTH 5
// 定义输出图像大小
#define OUTPUT_HEIGHT (INPUT_HEIGHT - KERNEL_SIZE + 1)
#define OUTPUT_WIDTH (INPUT_WIDTH - KERNEL_SIZE + 1)
// 定义卷积核
const int kernel[KERNEL_SIZE][KERNEL_SIZE] = {
{1, 2, 1},
{0, 0, 0},
{-1, -2, -1}
};
// 定义输入图像
const int input[INPUT_HEIGHT][INPUT_WIDTH] = {
{1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{11, 12, 13, 14, 15},
{16, 17, 18, 19, 20},
{21, 22, 23, 24, 25}
};
// 定义输出图像
int output[OUTPUT_HEIGHT][OUTPUT_WIDTH];
// img2col函数,将输入图像转换为矩阵形式
void img2col(int input[INPUT_HEIGHT][INPUT_WIDTH], int output[OUTPUT_HEIGHT * OUTPUT_WIDTH][KERNEL_SIZE * KERNEL_SIZE])
{
int row, col, kernel_row, kernel_col, index = 0;
for (row = 0; row < INPUT_HEIGHT - KERNEL_SIZE + 1; row++) {
for (col = 0; col < INPUT_WIDTH - KERNEL_SIZE + 1; col++) {
for (kernel_row = 0; kernel_row < KERNEL_SIZE; kernel_row++) {
for (kernel_col = 0; kernel_col < KERNEL_SIZE; kernel_col++) {
output[index][(kernel_row * KERNEL_SIZE) + kernel_col] = input[row + kernel_row][col + kernel_col];
}
}
index++;
}
}
}
// 矩阵乘法函数,计算卷积
void matrix_multiply(int input[OUTPUT_HEIGHT * OUTPUT_WIDTH][KERNEL_SIZE * KERNEL_SIZE], int kernel[KERNEL_SIZE][KERNEL_SIZE], int output[OUTPUT_HEIGHT][OUTPUT_WIDTH])
{
int row, col, i;
for (row = 0; row < OUTPUT_HEIGHT * OUTPUT_WIDTH; row++) {
int sum = 0;
for (i = 0; i < KERNEL_SIZE * KERNEL_SIZE; i++) {
sum += input[row][i] * kernel[i / KERNEL_SIZE][i % KERNEL_SIZE];
}
col = row % OUTPUT_WIDTH;
row /= OUTPUT_WIDTH;
output[row][col] = sum;
}
}
int main()
{
int input_matrix[OUTPUT_HEIGHT * OUTPUT_WIDTH][KERNEL_SIZE * KERNEL_SIZE];
img2col(input, input_matrix);
matrix_multiply(input_matrix, kernel, output);
// 输出结果
int i, j;
for (i = 0; i < OUTPUT_HEIGHT; i++) {
for (j = 0; j < OUTPUT_WIDTH; j++) {
printf("%d ", output[i][j]);
}
printf("\n");
}
return 0;
}
```
这里实现了一个3x3的卷积核对一个5x5的输入图像进行卷积,输出3x3的图像。首先将输入图像转换为矩阵形式,然后进行矩阵乘法计算卷积,最后输出结果。
相关推荐
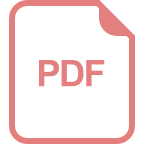
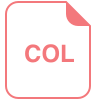
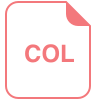
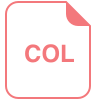
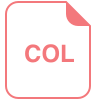
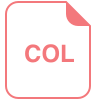









