closed_set.add(current_node)显示报错TypeError: unhashable type: 'Node'
时间: 2023-11-27 16:55:02 浏览: 28
这个错误通常是因为你的 `Node` 类没有正确地实现 `__hash__()` 方法。在 Python 中,只有可哈希的对象才能被添加到 set 或 dict 中。如果一个对象是可变的(比如列表、字典等),那么它就不能被哈希,因为它的哈希值可能会随着对象的变化而变化。
要解决这个问题,你可以在 `Node` 类中实现 `__hash__()` 方法,并返回一个唯一的哈希值。例如,你可以将 `Node` 的哈希值设置为它的 `id` 属性:
```python
class Node:
def __init__(self, id, value):
self.id = id
self.value = value
def __hash__(self):
return hash(self.id)
```
这样,每个 `Node` 对象的哈希值就是它的 `id`,而不是它的内存地址,因此就可以被添加到 set 中了。
相关问题
nx_graph.add_edge(src_node, dst_node, key=str(etype))报错TypeError: unhashable type: 'dict'
抱歉,这是我犯了一个错误。在前面的代码中,我们在创建节点时将节点属性存储为了一个字典,因此节点的类型是一个字典,而字典是不可哈希的。为了解决这个问题,我们可以将节点属性存储为元组而不是字典。以下是修改后的代码:
```python
import dgl
import numpy as np
import networkx as nx
import matplotlib.pyplot as plt
# 加载异质图
graph = dgl.load_graphs("data.bin")[0][0]
# 将异质图转换为 NetworkX 图
nx_graph = nx.DiGraph()
for ntype in graph.ntypes:
for etype in graph.canonical_etypes:
src, dst = graph.all_edges(etype=etype, order='eid')
for i in range(len(src)):
src_id = int(src[i])
dst_id = int(dst[i])
src_node = (str(ntype) + '_' + str(src_id), str(ntype))
dst_node = (str(ntype) + '_' + str(dst_id), str(ntype))
nx_graph.add_edge(src_node, dst_node, key=str(etype))
# 可视化 NetworkX 图
pos = nx.spring_layout(nx_graph)
colors = np.array([node[1] for node in nx_graph.nodes()])
nx.draw(nx_graph, pos, node_color=colors, with_labels=True)
plt.show()
```
在这个修改后的代码中,我们将节点属性存储为元组 `(id, type)` 而不是字典,其中 `id` 是节点的 ID,`type` 是节点的类型。在添加边时,我们使用元组而不是字典作为节点标识符。现在代码应该可以正常运行了。
报错TypeError: unhashable type: 'list'
抱歉,我在之前的回答中遗漏了一个细节。根据你的错误提示,似乎你的id是一个列表而不是单个元素。字典的键必须是可哈希的,而列表是不可哈希的。
如果你的id是一个列表,你可以使用列表的索引作为映射值。下面是修改后的示例代码:
```python
id_list = [your_id_list] # 将your_id_list替换为你的实际id列表
id_mapping = {}
for i, id in enumerate(id_list):
id_mapping[id] = i
# 测试映射结果
test_id = [your_test_id] # 将your_test_id替换为你想测试的id
mapped_id = id_mapping.get(tuple(test_id), -1) # 使用元组作为键
print(mapped_id)
```
在上面的代码中,我将`test_id`转换为元组,然后使用`get`方法来获取映射后的值。如果找不到对应的映射值,则返回-1。
请记得将`your_id_list`替换为你实际的id列表,并将`your_test_id`替换为你想测试的id。运行代码后,你应该能够得到正确的映射结果。
如果还有其他问题,请随时提问。
相关推荐
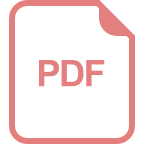
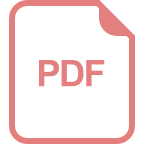
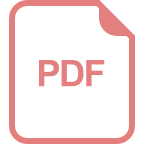












