给我详细讲一下这部分 do { for (int i = 0; i < n; i++) cout << c[i]<<" "; cout << endl; } while (next_permutation(c, c + n));
时间: 2024-04-25 10:26:57 浏览: 18
这段代码使用了C++ STL中的`next_permutation`函数来生成全排列。程序首先读入n个元素并存放在数组c中,然后对数组c进行排序,以便生成排列时按照升序的方式进行。
接下来使用了do-while循环,循环中使用了`next_permutation`函数,该函数会将当前的排列转换为下一个排列。`next_permutation`函数需要接收两个参数,第一个参数是需要进行排列的起始位置,第二个参数是需要进行排列的结束位置(不包括该位置)。
在每次循环中,程序会通过for循环输出当前排列,然后在使用`next_permutation`函数生成下一个排列,如果当前排列为最后一个排列,则`next_permutation`函数返回false,do-while循环终止,程序结束。
总的来说,这段代码使用了C++ STL中的`next_permutation`函数来实现了全排列的功能,代码简单易懂,易于实现。
相关问题
#include <algorithm> #include <iostream> using namespace std; int main() { int n; cin >> n; int* c = new int[n]; for (int i = 0; i < n; i++) cin >> c[i]; sort(c, c + n); do { for (int i = 0; i < n; i++) cout << c[i]<<" "; cout << endl; } while (next_permutation(c, c + n)); return 0; }不用do while怎么写
可以使用递归的方式来实现全排列,具体实现如下:
```
#include <algorithm>
#include <iostream>
using namespace std;
void permutation(int* arr, int start, int end)
{
if(start == end)
{
for(int i = 0; i <= end; i++)
cout << arr[i] << " ";
cout << endl;
}
else
{
for(int i = start; i <= end; i++)
{
swap(arr[start], arr[i]);
permutation(arr, start+1, end);
swap(arr[start], arr[i]);
}
}
}
int main()
{
int n;
cin >> n;
int* arr = new int[n];
for(int i = 0; i < n; i++)
cin >> arr[i];
sort(arr, arr + n);
permutation(arr, 0, n-1);
delete[] arr;
return 0;
}
```
这个程序与之前的程序有很大的相似性,只是将do-while循环改为了递归函数。程序先对输入的元素进行排序,然后调用递归函数permutation来进行全排列。递归终止条件是只剩下一个元素时,直接输出即可。
优化这段代码:#include<iostream> #include<cstdio> #include<algorithm> using namespace std; int n; string s[25]; bool cmp(string a,string b){ return a+b>b+a; } int main(){ cin>>n; for(int i=1;i<=n;i++){ cin>>s[i]; } sort(s+1,s+n+1,cmp); for(int i=1;i<=n;i++){ cout<<s[i]; } return 0; }
这段代码主要是实现对字符串数组进行排序,排序规则为将字符串连接起来,比较两个连接后的字符串大小,从而确定两个字符串的排序。
以下是针对这段代码的一些优化建议:
1. 尽量少使用std命名空间,可以使用using namespace std;语句,但是建议最好不要使用,而是使用std::来引用命名空间中的函数和变量。
2. 在排序时,可以使用快速排序算法,因为该算法的平均时间复杂度为O(nlogn),并且在实际应用中表现优秀。
3. 在字符串连接时,可以使用stringstream来将多个字符串连接成一个字符串,这样会更加高效。
优化后的代码如下:
```c++
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<sstream>
using namespace std;
const int MAXN = 25;
int n;
string s[MAXN];
bool cmp(string a,string b){
stringstream ss1, ss2;
ss1 << a << b;
ss2 << b << a;
return ss1.str() > ss2.str();
}
void quick_sort(int l, int r) {
if (l >= r) return;
int i = l - 1, j = r + 1;
string mid = s[(l + r) >> 1];
while (i < j) {
do i++; while (cmp(s[i], mid));
do j--; while (cmp(mid, s[j]));
if (i < j) swap(s[i], s[j]);
}
quick_sort(l, j), quick_sort(j + 1, r);
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
cin>>n;
for(int i=1;i<=n;i++){
cin>>s[i];
}
quick_sort(1, n);
for(int i=1;i<=n;i++){
cout<<s[i];
}
return 0;
}
```
在上述代码中,首先定义了一个MAXN常量,表示字符串数组的最大长度,方便后续的代码编写和调试。
其次,将输入和输出的速度优化为与C语言相同的速度,可以使用`ios::sync_with_stdio(false)`以及`cin.tie(0)`和`cout.tie(0)`语句来实现。
然后是排序函数的优化,这里使用了快速排序算法,排序规则使用了原来的cmp函数,在比较时使用stringstream来将多个字符串连接成一个字符串进行比较。
最后,调用快速排序函数进行排序,然后输出结果即可。
相关推荐
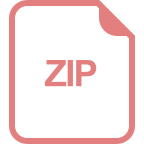
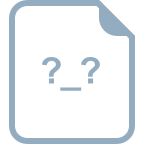
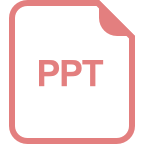












