爬取网页信息存储到doc格式文件,并写成函数,使用python代码
时间: 2023-08-31 20:24:55 浏览: 81
### 回答1:
下面是一个用 Python 爬取网页信息,并将其存储到 doc 格式文件的示例代码:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def scrape_and_save(url, file_name):
# 爬取网页
response = requests.get(url)
html = response.text
soup = BeautifulSoup(html, 'html.parser')
# 创建 docx 文件
document = Document()
# 在 docx 文件中添加爬取到的网页信息
document.add_paragraph(soup.prettify())
# 保存 docx 文件
document.save(file_name)
url = 'https://www.example.com'
file_name = 'example.docx'
scrape_and_save(url, file_name)
```
这段代码使用了 `requests` 库来爬取网页,使用 `BeautifulSoup` 库来解析 HTML 内容,使用 `docx` 库来创建和保存 docx 文件。
它会爬取给定的 `url` 地址的网页内容,然后将网页内容添加到一个新的 docx 文件中,最后将这个 docx 文件保存到给定的文件名 `file_name` 中。
希望这段代码能够帮到你!
### 回答2:
爬取网页信息并存储到doc格式文件,可以使用python的requests和BeautifulSoup库。下面是一个示例函数:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_save_to_doc(url, doc_file):
# 发送GET请求获取网页内容
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 创建一个doc文档对象
doc = Document()
# 在文档中添加标题
doc.add_heading('网页信息', 0)
# 获取网页标题
title = soup.title.string
# 在文档中添加网页标题
doc.add_heading(title, level=1)
# 获取网页正文内容
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
# 在文档中添加正文段落
doc.add_paragraph(paragraph.get_text())
# 保存文档
doc.save(doc_file)
# 测试
url = 'https://www.example.com'
doc_file = 'example.doc'
crawl_and_save_to_doc(url, doc_file)
```
以上函数通过向指定的URL发送GET请求获取网页内容,然后使用BeautifulSoup库解析html内容。接下来,创建一个doc文档对象,添加标题和网页标题,并获取网页的正文内容,添加到文档中。最后,将文档保存为doc格式文件。你可以将参数url和doc_file替换成自己需要的网页地址和文件名。另外,你可能需要在运行代码之前安装requests、BeautifulSoup和python-docx库。
### 回答3:
以下是使用Python编写的函数,用于爬取网页信息并将其保存为.doc格式文件:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_save_to_doc(url, file_name):
# 发送HTTP GET请求,获取网页内容
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 创建一个新的.doc文件
document = Document()
# 遍历所有的<p>标签,并将其内容添加到.doc文件中
for paragraph in soup.find_all('p'):
document.add_paragraph(paragraph.get_text())
# 保存.doc文件
document.save(file_name)
# 要爬取的网页URL
url = 'https://example.com'
# 保存为.doc文件的文件名
file_name = 'output.docx'
# 调用函数进行爬取并保存到.doc文件
crawl_and_save_to_doc(url, file_name)
```
使用这个函数,你可以将指定网页的所有`<p>`标签内容保存到名为`output.docx`的.doc文件中。你只需要将`url`变量替换为你要爬取的网页URL,并将`output.docx`替换为你要保存的.doc文件的文件名。
相关推荐
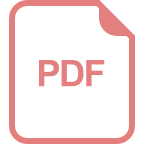
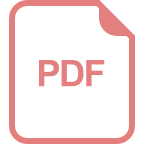














