openmv和stm32通信在将数据通过串口传到电脑上
时间: 2023-08-04 21:08:34 浏览: 104
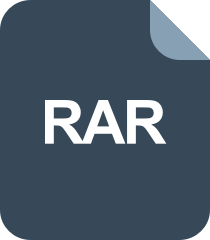
STM32与OpenMV串口通信实现

要实现 OpenMV 和 STM32 之间的通信,并将数据通过串口传输到电脑上,可以按照以下步骤进行:
1. 在 OpenMV 中,使用 `uart` 模块配置串口,并发送数据到串口:
```python
import pyb
# 配置串口
uart = pyb.UART(3, 115200, timeout_char=1000)
# 发送数据到串口
uart.write("Hello, STM32!\r\n")
```
在上述代码中,我们使用 `pyb.UART()` 函数初始化了一个串口对象,并将波特率设置为 115200。然后,我们使用 `uart.write()` 函数将数据发送到串口。
2. 在 STM32 中,使用 `USART` 模块初始化串口,并接收数据:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define USARTx USART1
#define USARTx_IRQn USART1_IRQn
#define USARTx_IRQHandler USART1_IRQHandler
void USARTx_IRQHandler(void)
{
static uint8_t rx_buffer[256];
static uint8_t rx_index = 0;
if(USART_GetITStatus(USARTx, USART_IT_RXNE) != RESET)
{
// 读取串口接收缓冲区数据
uint16_t data = USART_ReceiveData(USARTx);
// 将数据存入接收缓冲区
rx_buffer[rx_index++] = (uint8_t)data;
// 判断是否收到完整的数据包
if(rx_index >= 13 && rx_buffer[0] == 'H' && rx_buffer[1] == 'e' && rx_buffer[2] == 'l' && rx_buffer[3] == 'l' && rx_buffer[4] == 'o' && rx_buffer[5] == ',' && rx_buffer[6] == ' ' && rx_buffer[7] == 'S' && rx_buffer[8] == 'T' && rx_buffer[9] == 'M' && rx_buffer[10] == '3' && rx_buffer[11] == '2' && rx_buffer[12] == '!')
{
// 处理完整的数据包
printf("Received data: %s", rx_buffer);
// 清空接收缓冲区
rx_index = 0;
}
// 清除中断标志位
USART_ClearITPendingBit(USARTx, USART_IT_RXNE);
}
}
int main(void)
{
// 使能串口时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// 初始化串口 GPIO 引脚
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 初始化串口参数
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USARTx, &USART_InitStructure);
// 使能串口中断
USART_ITConfig(USARTx, USART_IT_RXNE, ENABLE);
NVIC_EnableIRQ(USARTx_IRQn);
// 启动串口
USART_Cmd(USARTx, ENABLE);
while(1)
{
// 主程序循环处理...
}
}
```
在上述代码中,我们初始化了一个名为 `rx_buffer` 的接收缓冲区,并在 `USARTx_IRQHandler()` 函数中实现了串口中断处理。当串口接收到数据时,我们将数据存入接收缓冲区,并判断是否收到完整的数据包。如果收到完整的数据包,则在函数中处理数据,并清空接收缓冲区。需要注意的是,在实际应用中,我们需要根据数据的格式和协议,来解析接收到的数据包。
3. 在 STM32 中,使用串口发送数据到电脑:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define USARTx USART1
int main(void)
{
// 使能串口时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// 初始化串口 GPIO 引脚
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 初始化串口参数
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USARTx, &USART_InitStructure);
// 启动串口
USART_Cmd(USARTx, ENABLE);
while(1)
{
// 发送数据到串口发送缓冲区
printf("Hello, PC!\r\n");
// 主程序循环处理...
}
}
```
在上述代码中,我们使用 `printf()` 函数将数据发送到串口发送缓冲区,并等待串口发送完毕。需要注意的是,为了使用 `printf()` 函数,我们需要在工程设置中开启 `printf()` 支持。
阅读全文
相关推荐
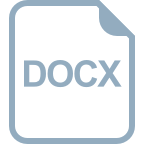
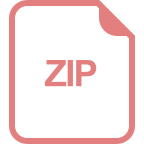
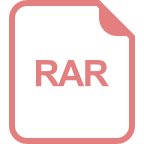
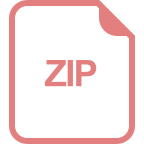
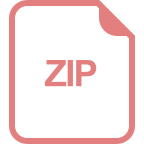
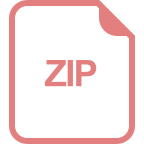
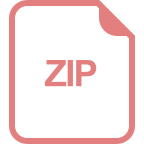
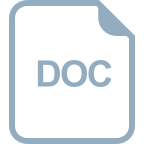
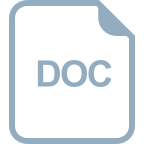
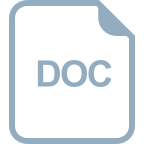
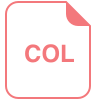





